1. Introduction
In this short tutorial, we're going to see how to configure Swagger UI to include a JSON Web Token (JWT) when it calls our API.
2. Maven Dependencies
In this example, we'll be using springfox-boot-starter, which includes all the necessary dependencies to start working with Swagger and Swagger UI. Let's add it to our pom.xml file:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>io.springfox</groupId>
<artifactId>springfox-boot-starter</artifactId>
<version>3.0.0</version>
</dependency>
3. Swagger Configuration
First, we need to define our ApiKey to include JWT as an authorization header:
private ApiKey apiKey() {
return new ApiKey("JWT", "Authorization", "header");
}
Next, let's configure the JWT SecurityContext with a global AuthorizationScope:
private SecurityContext securityContext() {
return SecurityContext.builder().securityReferences(defaultAuth()).build();
}
private List<SecurityReference> defaultAuth() {
AuthorizationScope authorizationScope = new AuthorizationScope("global", "accessEverything");
AuthorizationScope[] authorizationScopes = new AuthorizationScope[1];
authorizationScopes[0] = authorizationScope;
return Arrays.asList(new SecurityReference("JWT", authorizationScopes));
}
And then, we configure our API Docket bean to include API info, security contexts, and security schemes:
@Bean
public Docket api() {
return new Docket(DocumentationType.SWAGGER_2)
.apiInfo(apiInfo())
.securityContexts(Arrays.asList(securityContext()))
.securitySchemes(Arrays.asList(apiKey()))
.select()
.apis(RequestHandlerSelectors.any())
.paths(PathSelectors.any())
.build();
}
private ApiInfo apiInfo() {
return new ApiInfo(
"My REST API",
"Some custom description of API.",
"1.0",
"Terms of service",
new Contact("Sallo Szrajbman", "www.baeldung.com", "salloszraj@gmail.com"),
"License of API",
"API license URL",
Collections.emptyList());
}
4. REST Controller
In our ClientsRestController, let's write a simple getClients endpoint to return a list of clients:
@RestController(value = "/clients")
@Api( tags = "Clients")
public class ClientsRestController {
@ApiOperation(value = "This method is used to get the clients.")
@GetMapping
public List<String> getClients() {
return Arrays.asList("First Client", "Second Client");
}
}
5. Swagger UI
Now, when we start our application, we can access the Swagger UI at the http://localhost:8080/swagger-ui/ URL.
Here's a look at the Swagger UI with Authorize button:
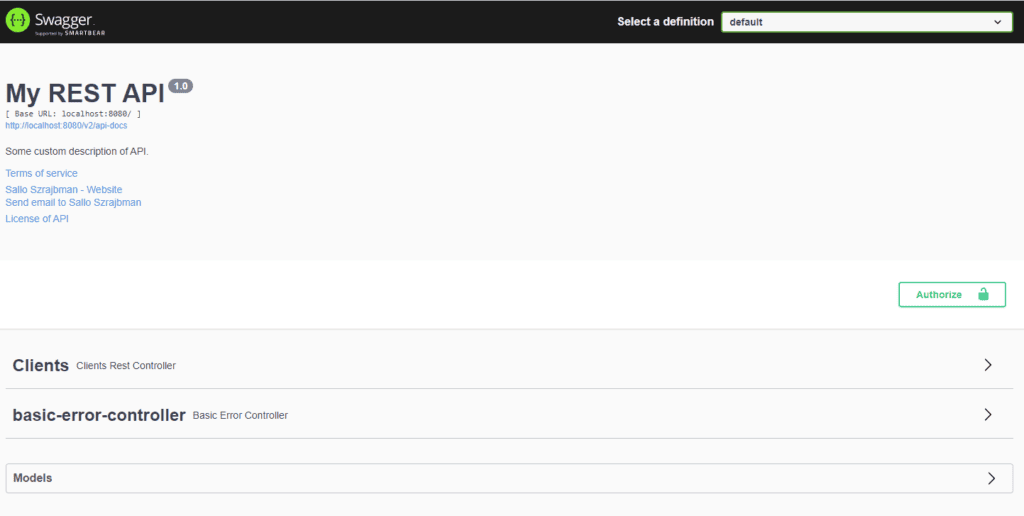
When we click the Authorize button, Swagger UI will ask for the JWT.
We just need to input our token and click on Authorize, and from then on, all the requests made to our API will automatically contain the token in the HTTP headers:
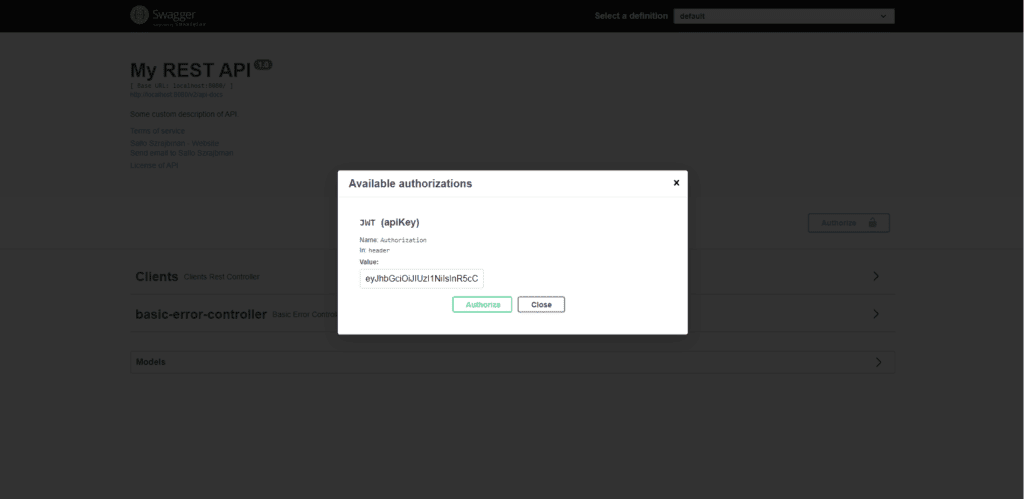
6. API Request with JWT
When sending the request to our API, we can see that there's an “Authorization” header with our token value:
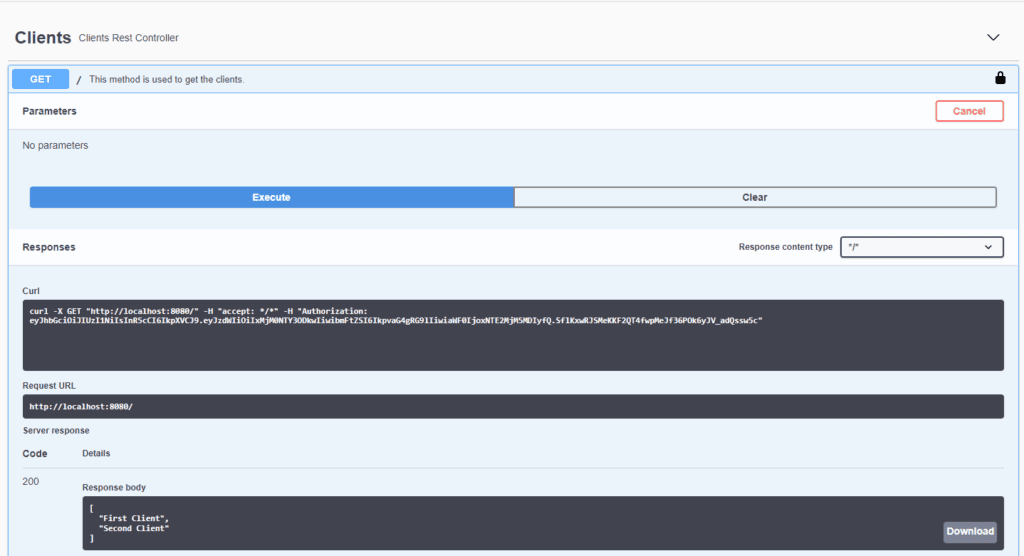
7. Conclusion
In this article, we saw how Swagger UI provides custom configurations to set up JWT, which can be helpful when dealing with our application authorization. After authorizing in Swagger UI, all the requests will automatically include our JWT.
The source code in this article is available over on GitHub.
The post Set JWT with Spring Boot and Swagger UI first appeared on Baeldung.