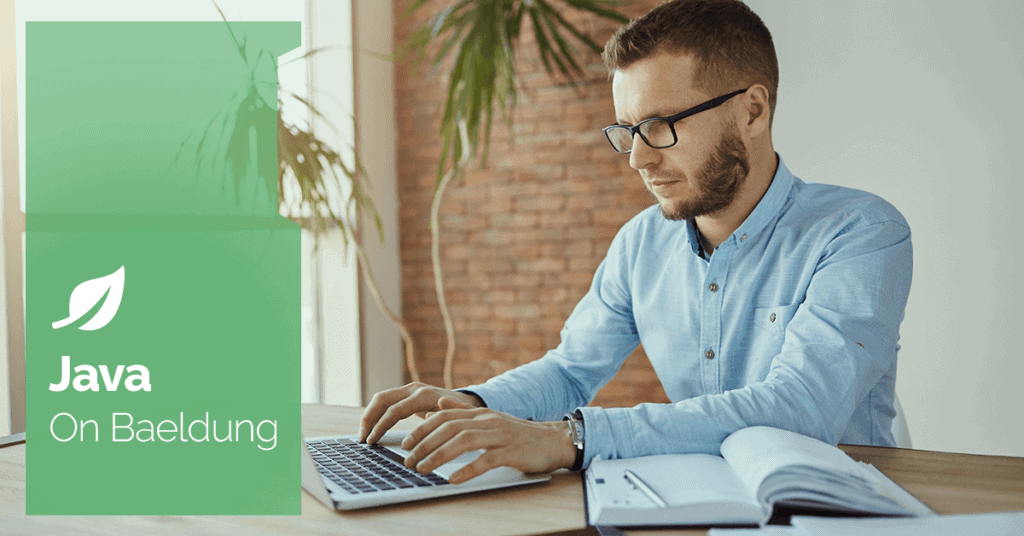
1. Overview
When we work with Java, dealing with arrays is a fundamental task. We often need to access the first and last elements of an array.
In this quick tutorial, we’ll explore how to efficiently get the first and last elements of an array in Java.
2. Short Introduction to Java Arrays
In Java, an array is a data structure that allows us to store a fixed-size sequence of elements.
The length property of an array returns the number of elements in the array. It’s important to note that length is a property, not a method, i.e., we access it without parentheses: array.length.
Random access in Java arrays is fast and efficient. Also, since Java arrays provide direct memory access to elements based on their indexes, accessing any element within the array has the same time complexity, regardless of the index.
Next, let’s see how to obtain the first and the last elements from an array.
3. Obtaining the First and the Last Elements
We’ve mentioned that it’s efficient to access elements in an array by indexes. Java arrays are zero-indexed, meaning the index of the first element is 0, the index of the second element is 1, and so on.
Therefore, we can use array[0] to access the first element in array. Moreover, we can determine the index of the last element by subtracting one from the length property: array.length – 1.
So next, let’s see an example of how to access the first and last elements from a String array:
String[] array = { "java", "kotlin", "c", "c#", "go", "python", "rust", "ruby" };
assertEquals("java", array[0]);
assertEquals("ruby", array[array.length - 1]);
As the example shows, we have a String array containing eight String values. We successfully obtained the first and the last elements using array[0] and array[array.length – 1].
4. What if the Array Is Empty?
We’ve learned how to retrieve the first and the last elements from an array using indexes. However, an array can be empty in Java.
Next, let’s see what happens if we access the first and the last elements from an empty array:
String[] array = new String[] {};
assertThrows(ArrayIndexOutOfBoundsException.class, () -> {String failing = array[0];});
assertThrows(ArrayIndexOutOfBoundsException.class, () -> {String failing = array[array.length - 1];});
The test above shows that accessing an empty array by indexes raises the ArrayIndexOutOfBoundsException.
A straightforward way to determine if an array is empty is to check whether its length equals 0:
String[] array = new String[] {};
assertEquals(0, array.length);
Therefore, it’s recommended to ensure the array isn’t empty before accessing its element:
if (array.length > 0){
// ... access elements by index
} else {
// ... handle the empty array case
}
The above example shows an if-else-based array empty check.
5. Conclusion
In this article, we’ve delved into retrieving the first and the last elements of an array:
- The first element – array[0]
- The last element – array[array.length – 1]
Accessing an array by its indexes is efficient. However, it’s imperative to verify that the array isn’t empty before attempting to retrieve elements by indexes.
As always, the complete source code for the examples is available over on GitHub.