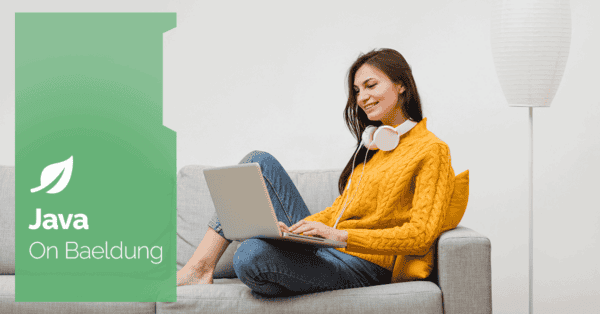
1. Overview
In this tutorial, we’ll explore how to perform drag-and-drop actions using Selenium and WebDriver. Drag-and-drop functionality is commonly used in web applications, from rearranging items on a page to handling file uploads. Automating this task with Selenium helps us cover user interaction.
We’ll discuss three core methods provided by Selenium: dragAndDrop(), clickAndHold(), and dragAndDropBy(). These methods allow us to simulate drag-and-drop actions with varying levels of control, from basic movements between elements to more complex, precise drags using offsets. Throughout the tutorial, we’ll demonstrate their usage on different test pages.
2. Setup and Configuration
Before getting started with drag-and-drop, we need to set up the environment. We’ll use the Selenium Java library and WebDriverManager to manage browser drivers. Our examples use Chrome but any other supported browser can be used similarly.
Let’s see the dependencies we need to include in our pom.xml:
<dependency>
<groupId>org.seleniumhq.selenium</groupId>
<artifactId>selenium-java</artifactId>
<version>4.27.0</version>
</dependency>
<dependency>
<groupId>io.github.bonigarcia</groupId>
<artifactId>webdrivermanager</artifactId>
<version>5.9.2</version>
</dependency>
Next, we initialize our WebDriver:
private WebDriver driver;
@BeforeEach
public void setup() {
driver = new ChromeDriver();
driver.manage().window().maximize();
}
@AfterEach
public void tearDown() {
driver.quit();
}
We’ll use a demo page to showcase our drag-and-drop examples.
3. Using the dragAndDrop() Method
In web development, elements are often made either draggable or droppable. A draggable element can be clicked and moved around the screen. On the other hand, a droppable element serves as a target where draggable elements can be placed. For example, in a task management application, we might drag a task (draggable) and drop it into another section (droppable) to organize tasks more efficiently.
Selenium’s dragAndDrop() method is a straightforward way to simulate dragging an element from one location to another, specifically from a draggable to a droppable. This method requires specifying the source (draggable) and the target (droppable) elements.
Let’s walk through how to implement it:
@Test
public void givenTwoElements_whenDragAndDropPerformed_thenElementsSwitched() {
String url = "http://the-internet.herokuapp.com/drag_and_drop";
driver.get(url);
WebElement sourceElement = driver.findElement(By.id("column-a"));
WebElement targetElement = driver.findElement(By.id("column-b"));
Actions actions = new Actions(driver);
actions.dragAndDrop(sourceElement, targetElement)
.build()
.perform();
}
In this test, we first navigate to the test page, locate the source and target elements by their IDs, and then perform the drag-and-drop action using dragAndDrop(). This method automatically handles the movement, making it suitable for simple use cases where we want to simulate basic drag-and-drop behavior.
4. Using the clickAndHold() Method
Sometimes, we need more control over the drag-and-drop operation. For instance, if we want to simulate hovering over an intermediate element or dragging slowly across the page, the clickAndHold() method gives us more granular control over each part of the drag sequence.
Here’s how we can implement it:
@Test
public void givenTwoElements_whenClickAndHoldUsed_thenElementsSwitchedWithControl() {
String url = "http://the-internet.herokuapp.com/drag_and_drop";
driver.get(url);
WebElement sourceElement = driver.findElement(By.id("column-a"));
WebElement targetElement = driver.findElement(By.id("column-b"));
Actions actions = new Actions(driver);
actions.clickAndHold(sourceElement)
.moveToElement(targetElement)
.release()
.build()
.perform();
}
In this case, we use clickAndHold() to click on the source element, moveToElement() to move it to the target, and release() to drop it. The flexibility of this approach allows us to simulate scenarios like dragging across a screen or interacting with elements in between.
Additional control is useful when testing features that require complex movements or multiple steps, such as dragging items into a specific section of a page or adjusting a slider.
5. Using dragAndDropBy() for Draggable Elements
In some cases, we may need to drag an element to an exact position rather than dropping it onto a target. The dragAndDropBy() method allows us to drag an element by a specific number of pixels along the X and Y axes. This is particularly useful for testing UI elements such as sliders or when working with resizable or moveable elements.
Let’s demonstrate this method using the jqueryui draggable demo page:
@Test
public void givenDraggableElement_whenDragAndDropByUsed_thenElementMovedByOffset() {
String url = "https://jqueryui.com/draggable/";
driver.get(url);
driver.switchTo().frame(0);
WebElement draggable = driver.findElement(By.id("draggable"));
Actions actions = new Actions(driver);
actions.dragAndDropBy(draggable, 100, 100)
.build()
.perform();
}
In this test, we first navigate to the jqueryui draggable demo page, switch to the iframe where the draggable element is located, and then use the dragAndDropBy() method to move the element by offset of 100 pixels along both X and Y axes.
The dragAndDropBy() method gives us the flexibility to move elements to any position on the page without the need for a specific droppable target. This can be particularly helpful when testing elements like sliders, panels, or image galleries, where precise movement is essential.
6. Conclusion
In this article, we discussed various methods to automate drag-and-drop functionality in Selenium. We started with the dragAndDrop() method, which moves elements between specified source and target locations. This method is ideal for scenarios involving draggable and droppable elements.
Next, we looked at the clickAndHold() method. This offers more control for complex movements like slow drags or interactions with intermediate elements. Finally, we talked about the dragAndDropBy() method, which allows for precise positioning of elements using offsets.
The complete code for these examples can be found over on GitHub.