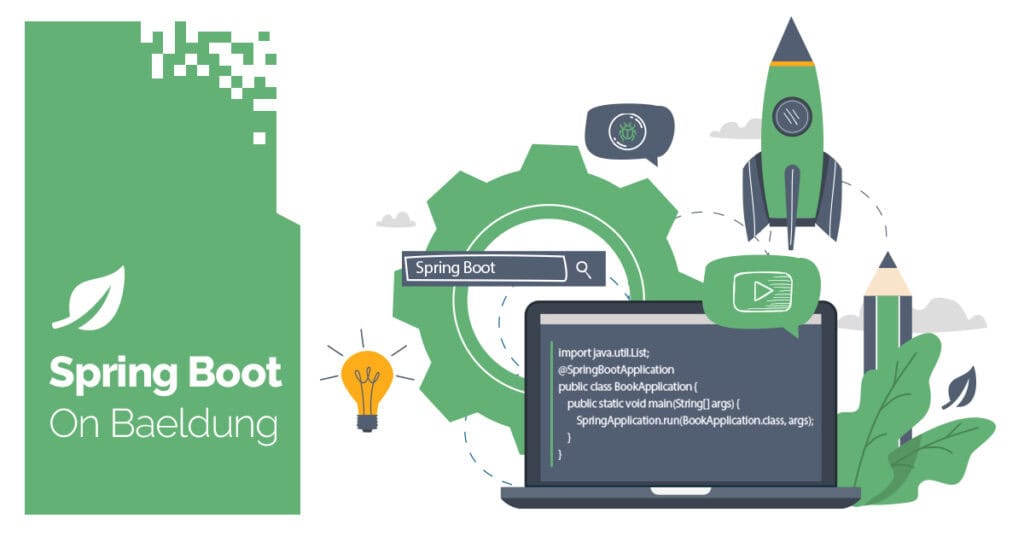
1. Overview
In distributed systems and microservices architectures, handling failures gracefully is crucial for maintaining system reliability and performance. Two fundamental resilience patterns that help achieve this are Circuit Breaker and Retry. While both patterns aim to improve system stability and reliability, they serve distinctly different purposes and are applied in different scenarios.
In this article, we’ll explore these patterns in depth, including their mechanisms, use cases, and implementation details using Resilience4j in Spring Boot.
2. What Is Retry?
The Retry pattern is a simple yet powerful mechanism that handles transient failures in distributed systems. When an operation fails, the Retry pattern attempts to execute the same operation multiple times, hoping that the temporary issue will resolve itself.
2.1. Key Characteristics of Retry
Retry mechanisms revolve around specific attributes that make them effective in handling transient issues, ensuring temporary glitches do not escalate into significant problems:
- Repeated attempts: The core idea is to re-execute a failed operation a specified number of times
- Backoff strategies: This is an advanced retry mechanisms that include backoff strategies, such as exponential backoff, which helps to avoid overwhelming the system
- Ideal for temporary failures: Best suited for intermittent network issues, temporary service unavailability, or momentary resource constraints
2.2. Retry Implementation Example
Let’s see a simple example of implementing a Retry mechanism using Resilience4j:
@Test
public void whenRetryWithExponentialBackoffIsUsed_thenItRetriesAndSucceeds() {
IntervalFunction intervalFn = IntervalFunction.ofExponentialBackoff(1000, 2);
RetryConfig retryConfig = RetryConfig.custom()
.maxAttempts(5)
.intervalFunction(intervalFn)
.build();
Retry retry = Retry.of("paymentRetry", retryConfig);
when(paymentService.process(1)).thenThrow(new RuntimeException("First Failure"))
.thenThrow(new RuntimeException("Second Failure"))
.thenReturn("Success");
Callable<String> decoratedCallable = Retry.decorateCallable(
retry, () -> paymentService.processPayment(1)
);
try {
String result = decoratedCallable.call();
assertEquals("Success", result);
} catch (Exception ignored) {
}
verify(paymentService, times(3)).processPayment(1);
}
In this example:
- The retry mechanism attempts the operation up to five times
- It employs an exponential backoff strategy to introduce a delay between attempts, reducing the risk of overloading the system
- The operation succeeds after two retries
3. What Is a Circuit Breaker Pattern?
The Circuit Breaker pattern is a more advanced approach to handling failures. It prevents an application from repeatedly trying to execute an operation that’s likely to fail, thereby preventing cascading failures and providing system stability.
3.1. Key Characteristics of Circuit Breaker
The Circuit Breaker pattern focuses on preventing excessive load on failing services and mitigating cascading failures. Let’s review its key attributes:
- State management: Circuit Breaker has three primary states:
- Closed: Normal Operation, allowing requests to proceed
- Open: Blocking all requests to prevent further failures
- Half-Open: Allowing a limited number of test requests to check if the system has recovered
- Failure threshold: Monitors the percentage of failed requests within a sliding window and “trips” the circuit when the failure rate exceeds the configured threshold
- Prevents cascading failures: Stops repeated calls to a failing service, protecting the entire system from degradation
3.2. Circuit Breaker Implementation Example
Here’s a simple example of Circuit Breaker implementation showcasing state transitions:
@Test
public void whenCircuitBreakerTransitionsThroughStates_thenBehaviorIsVerified() {
CircuitBreakerConfig circuitBreakerConfig = CircuitBreakerConfig.custom()
.failureRateThreshold(50)
.slidingWindowSize(5)
.permittedNumberOfCallsInHalfOpenState(3)
.build();
CircuitBreaker circuitBreaker = CircuitBreaker.of("paymentCircuitBreaker", circuitBreakerConfig);
AtomicInteger callCount = new AtomicInteger(0);
when(paymentService.processPayment(anyInt())).thenAnswer(invocationOnMock -> {
callCount.incrementAndGet();
throw new RuntimeException("Service Failure");
});
Callable<String> decoratedCallable = CircuitBreaker.decorateCallable(
circuitBreaker, () -> paymentService.processPayment(1)
);
for (int i = 0; i < 10; i++) {
try {
decoratedCallable.call();
} catch (Exception ignored) {
}
}
assertEquals(5, callCount.get());
assertEquals(CircuitBreaker.State.OPEN, circuitBreaker.getState());
callCount.set(0);
circuitBreaker.transitionToHalfOpenState();
assertEquals(CircuitBreaker.State.HALF_OPEN, circuitBreaker.getState());
reset(paymentService);
when(paymentService.processPayment(anyInt())).thenAnswer(invocationOnMock -> {
callCount.incrementAndGet();
return "Success";
});
for (int i = 0; i < 3; i++) {
try {
decoratedCallable.call();
} catch (Exception ignored) {
}
}
assertEquals(3, callCount.get());
assertEquals(CircuitBreaker.State.CLOSED, circuitBreaker.getState());
}
In this example:
- A 50% failure rate threshold and a sliding window of five calls determine when the circuit breaker “trips”.
- After five failed attempts, the circuit opens, immediately rejecting further calls.
- The circuit transitions to Half-Open after one one-second wait.
- In the Half-Open state, three successful calls are made, leading the circuit breaker to transition to Closed, resuming normal operations.
4. Key Differences: Retry vs. Circuit Breaker
Aspect | Retry Pattern | Circuit Breaker Pattern |
---|---|---|
Primary Goal | Attempts operation multiple times | Prevent repeated calls to a failing service |
Failure Handling | Assumes transient failures | Assumes potential Systemic failures |
State Management | Stateless, repeatedly attempts | Maintains state (Closed/Open/Half-Open) |
Best used For | Intermittent, recoverable errors | Persistent or systemic failures |
5. When to Use Each Pattern
Deciding when to use Retry or Circuit Breaker depends on the type of failures our system is encountering. These patterns complement each other, and understanding their application can help us build resilient systems that handle errors effectively.
- Use Retry when:
- Dealing with transient network issues
- Temporary service unavailability is expected
- Quick recovery is likely within a few retries
- Use Circuit Breaker when:
- Protecting against prolonged service failures
- Preventing cascading failures in microservices
- Implementing self-healing system architectures
In real-world applications, these patterns are often used together. For example, a Retry mechanism can work within the bounds of a Circuit Breaker, ensuring that retries are attempted only when the circuit is closed or half-open.
6. Best Practices
To maximize the effectiveness of these patterns:
- Monitor metrics: Continuously monitor failure rates, retry attempts, and circuit states to fine-tune configurations.
- Combine patterns: Use Retry for transient errors and Circuit Breaker for systemic failures.
- Set realistic thresholds: Overly aggressive thresholds can either hinder recovery or delay failure detection.
- Leverage libraries: Use robust libraries like Resilience4j or Spring Cloud Circuit Breaker, which implements both Resilience4j and Spring Retry under the hood, to simplify implementation.
7. Spring Boot Integration
Spring Boot offers comprehensive support for both Circuit Breaker and Retry patterns through its ecosystem. This integration is primarily achieved through the Spring Cloud Circuit Breaker project and Spring Retry module.
The Spring Cloud Circuit Breaker project provides an abstraction layer that allows us to implement circuit breakers without being tied to a specific implementation. This means we can switch between different circuit breaker implementations (like Resilience4j, Hysterix, Sentinel, or Spring Retry) based on our needs without changing our application code. The project uses Spring Boot’s auto-configuration mechanism, which automatically configures necessary circuit breaker beans when it detects the appropriate starter in the classpath.
For retry functionality, Spring Boot integrates with Spring Retry, offering both annotation-based and programmatic approaches to implementing retry logic. The framework provides flexible configuration options through both properties files and Java configuration, allowing us to customize retry attempts, backoff policies, and recovery strategies.
Let’s look at a few characteristics of Spring Boot’s integration with these patterns that make it particularly powerful:
- Auto-configuration support: Spring Boot automatically configures circuit breaker and retry beans based on the dependencies in our classpath, reducing boilerplate configuration code.
- Pluggable architecture: The abstraction layer allows us to switch between different circuit breaker implementations without modifying our business logic.
- Configuration flexibility: Both patterns can be configured through application properties or Java configuration, supporting both global and specific configurations for different services.
- Integration with Spring ecosystem: These patterns work seamlessly with other Spring components like RestTemplate, WebClient, and various Spring Cloud components.
- Monitoring and metrics: Spring Boot’s actuator integration provides built-in monitoring capabilities for circuit breakers and retry attempts, helping us track the health and behavior of our resilience mechanisms.
This integration approach aligns with Spring Boot’s philosophy of convention over configuration while maintaining the flexibility to customize behavior when needed. The framework’s support for these patterns makes it easier to build resilient microservices that can handle failures gracefully and maintain system stability.
8. Conclusion
Both Retry and Circuit Breaker are essential resilience patterns in distributed systems. While Retry focuses on immediate recovery, Circuit Breaker provides robust protection against cascading failures. By understanding their differences and use cases, we can design systems that are both reliable and fault-tolerant.
With libraries like Resilience4j and Spring Cloud Circuit Breaker, Spring Boot offers a powerful platform to implement these patterns effortlessly. By adopting these resilience strategies, we can build applications capable of withstanding failures gracefully, ensuring a seamless user experience even in adverse conditions.
As usual, the complete code with all the examples presented in this article is available over on GitHub.
The post Difference Between Circuit Breaker and Retry in Spring Boot first appeared on Baeldung.