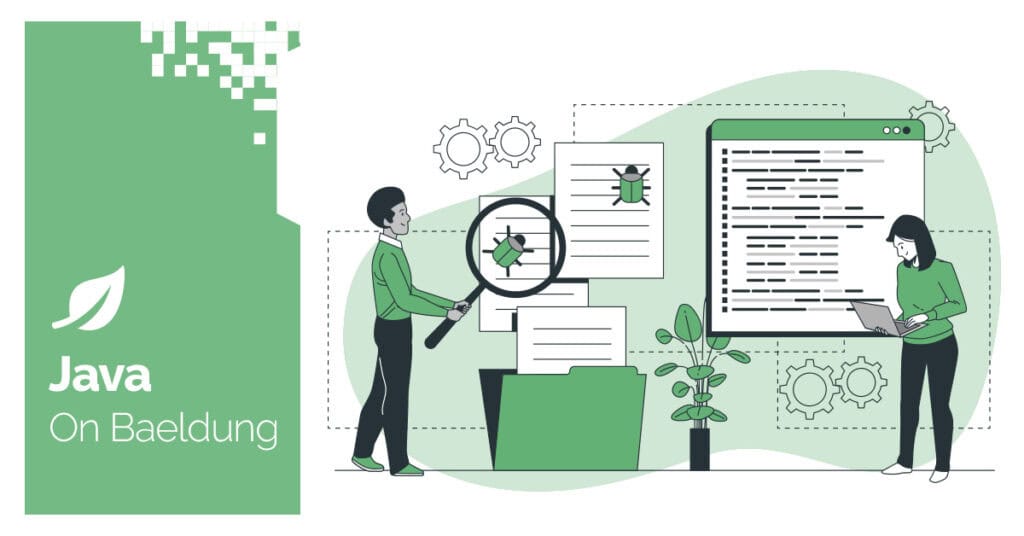
1. Overview
Mocking is a very useful feature in unit testing, especially in isolating the single behavior we want to test in our method. In this tutorial, we’ll see how we can mock a method in the same test class, using Mockito Spy.
2. Understanding the Problem
Unit testing is the first level of testing and our first defense against bugs. However, sometimes a method is too complex with multiple calls, especially in legacy code, where we don’t always have the possibility for refactoring. To simplify unit testing, we’ll demonstrate the power of Mockito Spy using a simple example.
For our example, let’s introduce a class CatTantrum:
public class CatTantrum {
public enum CatAction {
BITE,
MEOW,
VOMIT_ON_CARPET,
EAT_DOGS_FOOD,
KNOCK_THING_OFF_TABLE
}
public enum HumanReaction {
SCREAM,
CRY,
CLEAN,
PET_ON_HEAD,
BITE_BACK,
}
public HumanReaction whatIsHumanReaction(CatAction action){
return switch (action) {
case MEOW -> HumanReaction.PET_ON_HEAD;
case VOMIT_ON_CARPET -> HumanReaction.CLEAN;
case EAT_DOGS_FOOD -> HumanReaction.SCREAM;
case KNOCK_THING_OFF_TABLE -> HumanReaction.CRY;
case BITE -> biteCatBack();
};
}
public HumanReaction biteCatBack() {
// Some logic
return HumanReaction.BITE_BACK;
}
}
The method whatIsHumanReaction() contains some logic and a call to the method biteCatBack(). We’ll be focusing on unit testing the method whatIsHumanReaction().
3. Solution Using Mockito Spy
Let’s write our unit test using Mockito Spy :
@Test public void givenMockMethodHumanReactions_whenCatActionBite_thenHumanReactionsBiteBack(){ // Given CatTantrum catTantrum = new CatTantrum(); CatTantrum catTantrum1 = Mockito.spy(catTantrum); Mockito.doReturn(HumanReaction.BITE_BACK).when(catTantrum1).biteCatBack(); // When HumanReaction humanReaction1 = catTantrum1.whatIsHumanReaction(CatAction.BITE); // Then assertEquals(humanReaction1, HumanReaction.BITE_BACK); }
The first step is to create a spy for our test object using Mockito.spy(catTantrum). This line allows us to mock the return value of the method biteCatBack() called by whatIsHumanReaction().
Notably, when we call the method we want to test, we need to call it on the spy object catTantrum1 and not the original object catTantrum.
Mockito spy allows us to use some methods directly from the class and to stub other methods. If we’d used a mock, we would’ve needed to stub all the method calls. On the other hand, if we’d used a real instance of an object, we wouldn’t be able to mock any method from that class.
In the example above, Mockito Spy allowed us to call, on the same object catTantrum1, the real method whatIsHumanReaction(), and to call a stub of method biteCatBack().
4. Conclusion
In this article, we learned how to use Mockito Spy to solve the issue of mocking a method in the same test class.
We should keep in mind that it’s generally better to write simpler, one-purpose methods. But if that’s not possible, the usage of Mockito Spy can help bypass this complexity.
The complete source code for the examples is available over on GitHub.
The post Mocking a Method in the Same Test Class Using Mockito Spy first appeared on Baeldung.