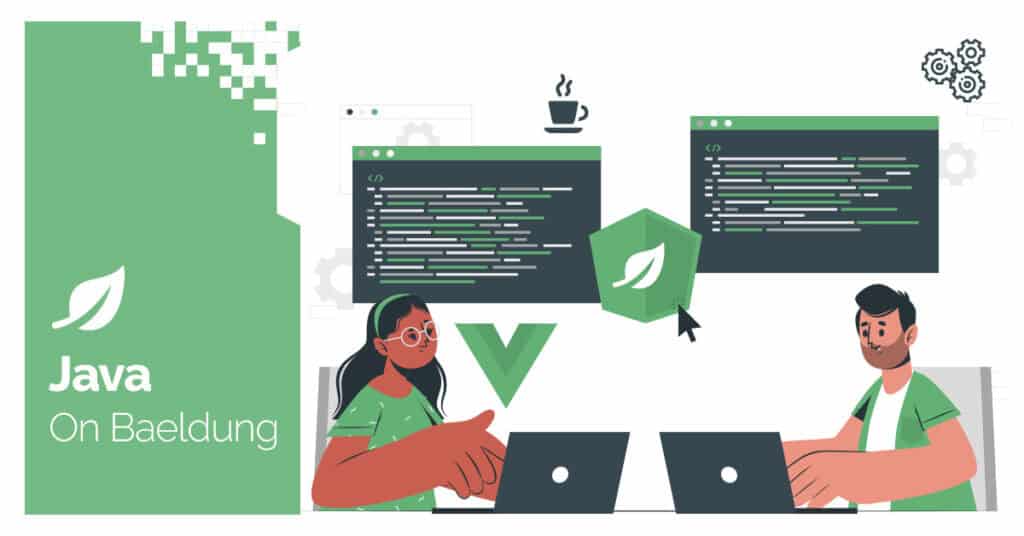
1. Overview
As Java developers, we’ve all faced compile-time errors, and one that might trip us up is “class X is public should be declared in a file named X.java”
In this quick tutorial, we’ll explain what this error means, why it happens, and how we can swiftly resolve it.
2. Introduction to the Problem
As usual, let’s understand the problem through examples.
Let’s say we want to create a simple football game application. So, first, let’s model players and clubs association in the FootballGame.java source file:
// Filename: FootballGame.java
public class FootballPlayer {
private String name;
private Club club;
public FootballPlayer(String name, Club club) {
this.name = name;
this.club = club;
}
// ... standard getters and setters are omitted
}
class Club {
private String name;
public Club(String name) {
this.name = name;
}
//... getters and setters are omitted
}
As the code shows, the two classes are pretty straightforward. However, when we compile this code, the compiler complains:
java: class FootballPlayer is public, and should be declared in a file named FootballPlayer.java
This compiler error can be confusing, particularly for Java beginners. Next, let’s understand what this error means and how to fix the issue.
3. Understanding the Error
First, in Java, a source file (*.java) can contain multiple classes but only one public class. In our example, we have FootballPlayer and Club in one file. Only the FootballPlayer class is public. So, everything looks fine.
However, whenever we declare a public class, we must ensure the filename exactly matches the name of the class. It’s Java’s way of enforcing structure and consistency in our code. Our public class is named FootballPlayer, but the filename is FootballGame.java. Therefore, we broke this rule.
Now that we understand the meaning of this compiler error. Next, let’s fix the problem.
4. How to Fix It
There are two different ways to fix this issue. Let’s examine each one.
4.1. Renaming the File
The error message says,” … FootballPlayer .. should be declared in a file named FootballPlayer.java“. So, the first fix is to rename the file to the public class name. In this example, we need to rename the file to FootballPlayer.java.
After renaming the file, the code is compiled without any error. Also, we can create a small test to verify if the two classes work as expected:
Club manUnited = new Club("Manchester United F.C.");
FootballPlayer rooney = new FootballPlayer("Wayne Rooney", manUnited);
assertEquals("Wayne Rooney", rooney.getName());
assertEquals("Manchester United F.C.", rooney.getClub().getName());
So, the problem is solved. Ensuring our filenames and public class names match, we align with Java’s conventions, making our code more organized and easier to manage.
Next, let’s move to an alternative solution.
4.2. Removing the public Modifier
We know that the root cause of this error is the mismatch between the filename (FootballGame.java) and the public class name (FootballPlayer). Another solution is to remove the public modifier from the FootballPlayer class:
// Filename: FootballGame.java
class FootballPlayer {
// ... same code is omitted
}
class Club {
// ... same code is omitted
}
As we don’t have a public class in this file, it eliminates the need for the filename to match the class name. Now, if we run the same test, the code compiles, and the test passes:
Club manUnited = new Club("Manchester United F.C.");
FootballPlayer rooney = new FootballPlayer("Wayne Rooney", manUnited);
assertEquals("Wayne Rooney", rooney.getName());
assertEquals("Manchester United F.C.", rooney.getClub() .getName());
The issue gets fixed. However, it’s important to note that by removing the public modifier, we make the class package-private (accessible only within the same package). For example, the test class above and the FootballGame.java file must be in the same package.
5. Conclusion
In this article, we have understood the compiler error “class X is public, should be declared in a file named X” and explored two different approaches to solving the issue.
When we reencounter this error, we’ll know exactly how to tackle it. With some practice, errors like this will become easy fixes in our development journey.
As always, the complete source code for the examples is available over on GitHub.
The post Fix The Compile Error: class X is public should be declared in a file named X.java first appeared on Baeldung.