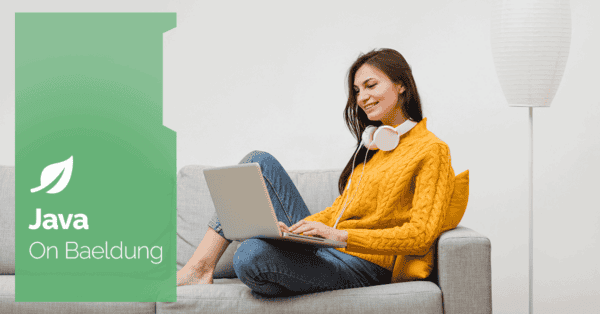
1. Introduction
In this tutorial, we’ll explore the importance of configuring connection/read timeouts in REST clients. We’ll demonstrate this using Jersey, a common JAX-RS implementation.
2. Why Set Connection and Read Timeouts?
Timeout settings for sockets are vital in applications where responsiveness and reliability are critical. For example, delays can impact user experience or result in transaction failures in financial or e-commerce applications.
Similarly, a misconfigured timeout in distributed systems can cause cascading delays and resource bottlenecks. Choosing appropriate timeout values ensures our application remains robust and responsive even in challenging network conditions.
3. Dependencies and Basic Setup
To understand how timeouts work, we’ll configure a Jersey client to call a slow REST API and observe the behavior when the response takes longer than the configured timeout.
3.1. Dependencies
For the client side, we’ll need jersey-client for HTTP communication with the API:
<dependency>
<groupId>org.glassfish.jersey.core</groupId>
<artifactId>jersey-client</artifactId>
<version>3.1.9</version>
</dependency>
For the server, we’ll need jersey-server:
<dependency>
<groupId>org.glassfish.jersey.core</groupId>
<artifactId>jersey-server</artifactId>
<version>3.1.9</version>
</dependency>
Then, for running the server in tests, we’ll need jaxrs-ri, jersey-container-grizzly2-servlet, and jersey-test-framework-provider-grizzly2:
<dependency>
<groupId>org.glassfish.jersey.bundles</groupId>
<artifactId>jaxrs-ri</artifactId>
<version>3.1.9</version>
</dependency>
<dependency>
<groupId>org.glassfish.jersey.containers</groupId>
<artifactId>jersey-container-grizzly2-servlet</artifactId>
<version>3.1.9</version>
</dependency>
<dependency>
<groupId>org.glassfish.jersey.test-framework.providers</groupId>
<artifactId>jersey-test-framework-provider-grizzly2</artifactId>
<version>3.1.9</version>
<scope>test</scope>
</dependency>
3.2. REST API Server
The REST API contains a single endpoint we’ll use in our tests. The operation’s implementation is irrelevant as we aim to simulate a delayed response. We’ll introduce some sleep time to simulate a slow server:
@Path("/timeout")
public class TimeoutResource {
public static final long STALL = TimeUnit.SECONDS.toMillis(2l);
@GET
public String get() throws InterruptedException {
Thread.sleep(STALL);
return "processed";
}
}
Later, we’ll use the STALL variable to determine our timeout values.
3.3. Client Setup
Our client only needs the endpoint URI and a general get(Client) method to access it. Let’s also define our TIMEOUT as half the STALL variable we defined earlier so we can enforce a timeout scenario:
public class JerseyTimeoutClient {
private static final long TIMEOUT = TimeoutResource.STALL / 2;
private final String endpoint;
public JerseyTimeoutClient(String endpoint) {
this.endpoint = endpoint;
}
private String get(Client client) {
return client.target(endpoint)
.request()
.get(String.class);
}
// ...
}
3.4. Test Setup
We’ll define two clients, one to test read timeouts and another to test connection timeouts.
Let’s start with the endpoint’s base address:
static final URI BASE = URI.create("http://localhost:8082");
To test read timeouts, we’ll use the correct endpoint. Since our timeout is half the time the server takes to process requests, a SocketTimeoutException is guaranteed:
static final String CORRECT_ENDPOINT = BASE + "/timeout";
JerseyTimeoutClient readTimeoutClient = new JerseyTimeoutClient(CORRECT_ENDPOINT);
Then, to test connection timeouts, we’ll replace the host with an unreachable IP address:
static final String INCORRECT_ENDPOINT = BASE.toString()
.replace(BASE.getHost(), "10.255.255.1");
JerseyTimeoutClient connectTimeoutClient = new JerseyTimeoutClient(INCORRECT_ENDPOINT);
Finally, let’s add an assertion method to reuse in our tests. It starts by catching the ProcessingException thrown by JAX-RS, and then we check the cause, which should always be a SocketTimeoutException. In the end, we check the message to differentiate between connection and read timeouts:
private void assertTimeout(String message, Executable executable) {
ProcessingException exception = assertThrows(ProcessingException.class, executable);
Throwable cause = exception.getCause();
assertInstanceOf(SocketTimeoutException.class, cause);
assertEquals(message, cause.getMessage());
}
Now that we’re ready to test, let’s see how to configure timeouts.
4. Using the ClientBuilder API
Let’s go back to JerseyTimeoutClient and add our first implementation using ClientBuilder. We can set both timeout configurations (connectTimeout() and readTimeout()) for the same client. We call the endpoint by passing our newly created client to the get() method we created earlier:
public String viaClientBuilder() {
ClientBuilder builder = ClientBuilder.newBuilder()
.connectTimeout(TIMEOUT, TimeUnit.MILLISECONDS)
.readTimeout(TIMEOUT, TimeUnit.MILLISECONDS);
return get(builder.build());
}
Let’s test the read timeout:
@Test
void givenCorrectEndpoint_whenClientBuilderAndSlowServer_thenReadTimeout() {
assertTimeout("Read timed out", readTimeoutClient::viaClientBuilder);
}
Now for the connection timeout test:
@Test
void givenIncorrectEndpoint_whenClientBuilder_thenConnectTimeout() {
assertTimeout("Connect timed out", connectTimeoutClient::viaClientBuilder);
}
All clients generated with this builder set both timeouts to the desired values. If not set, the default behavior is to wait indefinitely for a response, which is the same as setting them to zero.
Next, based on different requirements, let’s explore three alternative methods for setting these timeouts on a Client.
5. Using the ClientConfig Object
We can also configure timeouts via the ClientConfig, and we can use it when building a new client:
public String viaClientConfig() {
ClientConfig config = new ClientConfig();
config.property(ClientProperties.CONNECT_TIMEOUT, TIMEOUT);
config.property(ClientProperties.READ_TIMEOUT, TIMEOUT);
return get(ClientBuilder.newClient(config));
}
Using the ClientConfig is helpful if we want to apply the same configuration to many clients. This is especially handy when we have many complex, dynamic configurations that we want to make reusable.
6. Using the client.property() Method
We can also set these properties directly on the Client:
public String viaClientProperty() {
Client client = ClientBuilder.newClient();
client.property(ClientProperties.CONNECT_TIMEOUT, TIMEOUT);
client.property(ClientProperties.READ_TIMEOUT, TIMEOUT);
return get(client);
}
This is necessary for Jersey versions before 2.1 because connectTimeout() for the ClientBuilder was unavailable.
7. Setting Timeouts Per-Request
Finally, let’s include a method that sets the timeout on the request using the property() method. We’ll start by overriding our get() method to include a timeout parameter:
private String get(Client client, Long requestTimeout) {
Builder request = client.target(endpoint).request();
if (requestTimeout != null) {
request.property(ClientProperties.CONNECT_TIMEOUT, requestTimeout);
request.property(ClientProperties.READ_TIMEOUT, requestTimeout);
}
return request.get(String.class);
}
Now, we can pass the desired request timeout value:
public String viaRequestProperty() {
return get(ClientBuilder.newClient(), TIMEOUT);
}
We use this setup to override global settings for a specific request or have variable demands for timeout values.
8. Conclusion
In this article, we learned how configuring the connection and read timeouts is crucial for building robust and responsive REST clients. By understanding the different approaches provided by Jersey, such as ClientBuilder, ClientConfig, and per-request configurations, we can tailor our client behavior to meet specific application needs.
As always, the source code is available over on GitHub.
The post Setting Connection Timeout and Read Timeout for Jersey first appeared on Baeldung.