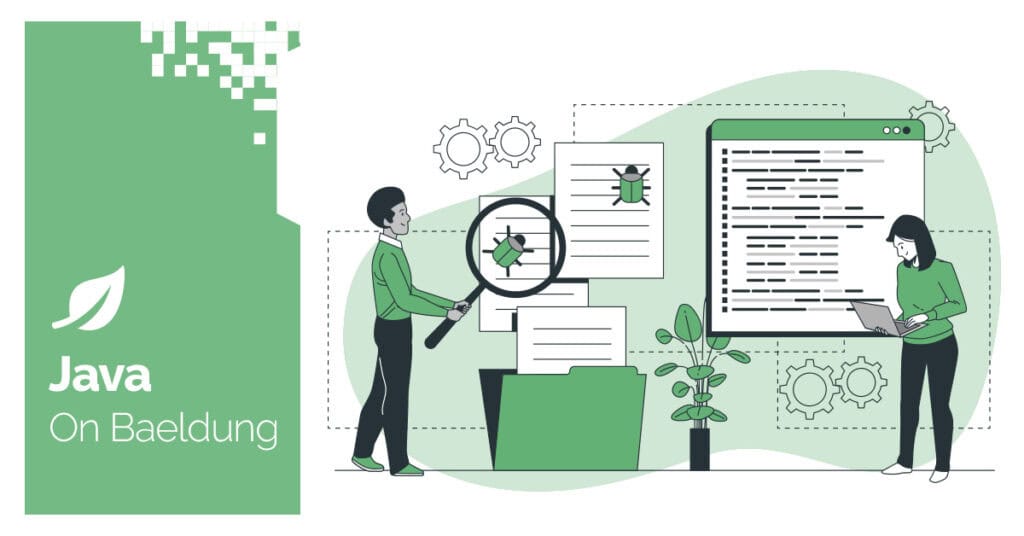
1. Overview
In Java programming, simplicity often hides behind the handling of null values, especially when dealing with arrays. Likewise, converting a potentially null array to an empty List isn’t just about preventing a NullPointerException; it’s also about crafting resilient code.
In this tutorial, we’ll discuss multiple approaches to transform a nullable array into a List safely.
2. Setup
First, let’s define our method signature, which we’ll use for our implementations:
static List<String> getAsList(String[] possiblyNullArray) {
// Implementation: return nullable array as list
}
We’ll accept a possiblyNullArray as our method argument, and return a non-null list that contains all the values from the original array. Importantly, if the original array is null or empty, our final list will be empty.
Now, let’s look at a few approaches.
3. Using Java 8+ Streams
In our first implementation, we’ll use the Java Streams API. Java 8 introduced the Optional class to deal with possibly missing or null values.
Let’s use Optional.ofNullable() first to deal with this possibly null array:
return Optional.ofNullable(possiblyNullArray)
.map(Arrays::stream)
.orElseGet(Stream::empty)
.collect(Collectors.toList());
We wrap our array in an Optional and then attempt to stream() the values. If the array is null, we’ll get an empty stream instead of the stream of values. This ensures that when we collect() the steam to a list, our result is a list of values from the array or an empty list.
If we want to avoid streaming the values, we can handle the default value from the Optional a little differently:
return Arrays.asList(Optional.ofNullable(possiblyNullArray).orElse(new String[0]));
Here, we’ll return an empty array if the original array is null before converting the array to a list.
Both approaches above safely return a List of Strings with a single expression.
4. Using Ternary Operator
Before Java 8, we can also use a simple ternary operator to check for null and return an empty List when our array is null:
return possiblyNullArray == null ? new ArrayList<>() : Arrays.asList(possiblyNullArray);
The example above is a short if/else expression: if the array is null, return an empty List, otherwise use Arrays.asList() – a simple and elegant solution.
5. Using Apache Commons Lang
Sometimes we might want to use an external dependency to do the work for us. The third implementation looks outside the core Java API and uses Apache Commons Lang.
This library provides an ArrayUtils class which includes nullToEmpty() which converts a null array to an empty one:
String[] notNullArray = ArrayUtils.nullToEmpty(possiblyNullArray);
return Arrays.asList(notNullArray);
Afterward, all that is left is to convert the array to a list.
6. Validation
Now that we’ve seen all our different implementations, let’s run each implementation against the following three tests:
@Test
public void whenArrayIsNull_thenReturnEmptyList() {
String[] possiblyNullArray = null;
assertThat(getAsList(possiblyNullArray)).isNotNull().isEmpty();
}
@Test
public void whenArrayIsEmpty_thenReturnEmptyList() {
String[] possiblyNullArray = {};
assertThat(getAsList(possiblyNullArray)).isNotNull().isEmpty();
}
@Test
public void whenArrayIsNotEmpty_thenReturnListWithSameElements() {
String[] possiblyNullArray = {"a", "b"};
assertThat(getAsList(possiblyNullArray)).containsExactly(possiblyNullArray);
}
With these three scenarios, we cover a null array, an empty array, and an array with some values, all possibilities for this problem.
7. Conclusion
In this article, we’ve learned how to convert a nullable array to a List with several approaches while avoiding possible NullPointerExceptions. We looked at the implementations with Java 8+ Optional capabilities, the ternary operator, and finally using external libraries like Apache Commons.
As always, the entire code used in this article can be found over on GitHub.
The post Null Array to Empty List in Java first appeared on Baeldung.