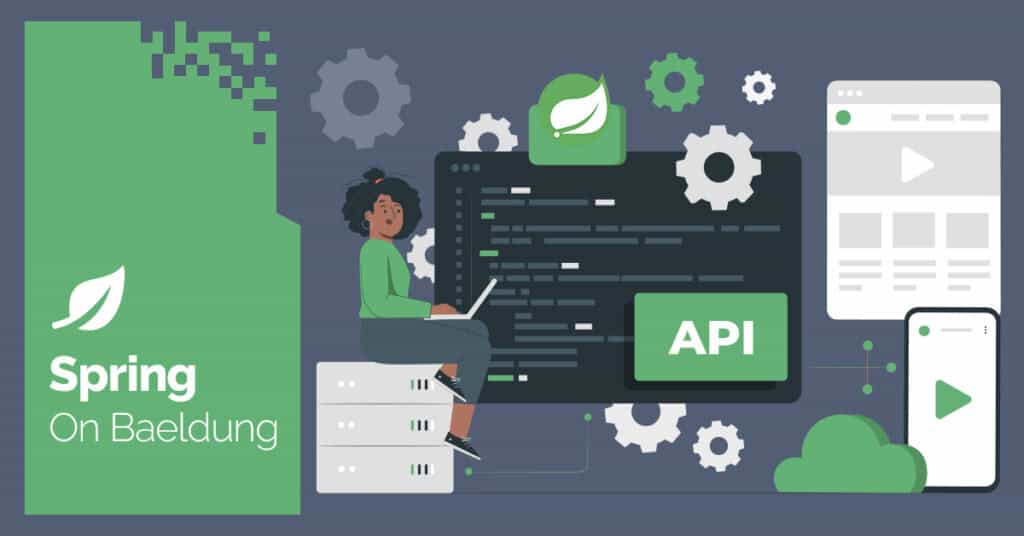
1. Overview
The process of validating various values is critical in software development. It ensures that the data flowing into our application is correct and consistent. Validations can be applied to any data type based on different factors.
In Spring, validation is streamlined through built-in tools and annotations, allowing us to implement robust validation logic easily.
In this tutorial, we’ll learn how to validate a list of values in Spring. We’ll also look at a case where only specific values are permitted. By leveraging standard and custom validation approaches, we can maintain data integrity and ensure seamless functionality across our application.
2. Setting up Validation in Spring
To enable validation, ensure we have the following dependency in our pom.xml:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-validation</artifactId>
</dependency>
This dependency integrates Hibernate Validator into our Spring application.
3. Validating a List of Values
Suppose we need to validate a list of employee roles. Each role should start with ROLE_.
So, at first, we add a variable for roles in the Employee DTO class:
@NotEmpty(message = "Roles list cannot be empty")
@Valid
private List<@Pattern(regexp = "ROLE_[A-Z]+",
message = "Each role must start with 'ROLE_' and contain uppercase letters only") String> roles;
The @NotEmpty annotation ensures the list isn’t empty and the @Pattern annotation validates that each role matches the specified format.
The next step is to add a method to the controller class so that we can trigger our validations:
@PostMapping("/validateListAtService")
public ResponseEntity<String> validateRoles(@RequestBody @Validated Employee request) {
return ResponseEntity.ok("Roles are valid!");
}
Here, the @Validated annotation ensures Spring performs validation on the Employee object.
At last, we can have a global exception handler class to handle validation errors:
@ExceptionHandler(MethodArgumentNotValidException.class)
@ResponseStatus(value = HttpStatus.BAD_REQUEST)
public String handleValidationException(MethodArgumentNotValidException ex) {
return ex.getBindingResult()
.getFieldErrors()
.stream()
.map(e -> e.getDefaultMessage())
.collect(Collectors.joining(","));
}
Let’s understand the annotations:
- The @ExceptionHandler annotation specifies the type of exception the method handles. In this case, it handles MethodArgumentNotValidException, which is thrown when validation on a method argument annotated with @Valid or @Validated fails
- The @ResponseStatus sets the HTTP status code for the response. Here, it sends a 400 BAD REQUEST status, indicating that the client provided invalid data
- The MethodArgumentNotValidException exception provides details about the validation errors, including the fields that failed validation and the associated error messages
Now, let’s discuss one of the scenarios where a validation error could occur.
Let’s say a client sends a request with invalid data:
{
"roles": ["admin", "ROLE_ADMIN,", ""]
}
As the validation rules specify that roles must start with ROLE_ and cannot be empty, the response look like this:
Each role must start with 'ROLE_' and contain uppercase letters only
The key benefit of having a global exception handler is that it ensures all validation errors are handled consistently. It provides user-friendly feedback to the client and it reduces the boilerplate by eliminating the need for error-handling logic in individual controllers.
4. Restricting Values to a Predefined List
Till now, we have seen one way of validating the list of values. Let’s consider another scenario where we want to input various departments of a specific employee. To implement this functionality, we’ll create a custom annotation that validates that the list of departments entered is valid, i.e., it’s part of a pre-defined list.
At first, we create a custom validator annotation:
@Constraint(validatedBy = AllowedValuesValidator.class)
@Target({ ElementType.FIELD })
@Retention(RetentionPolicy.RUNTIME)
public @interface AllowedValues {
String message() default "Invalid value";
String[] values();
Class<?>[] groups() default {};
Class<? extends Payload>[] payload() default {};
}
Let’s understand this code:
- @Constraint: Specifies the class (AllowedValuesValidator) responsible for validating the annotation’s logic
- @Target: Restricts where this annotation can be applied (e.g., fields or method parameters)
- @Retention: Ensures the annotation is available at runtime for validation
- values: Accepts a predefined set of allowed values for validation
- message: Custom error message if validation fails
- groups and payload: Optional attributes for grouping constraints and custom metadata
The next step is to implement our validator:
public class AllowedValuesValidator implements ConstraintValidator<AllowedValues, List<String>> {
private List<String> allowedValues;
@Override
public void initialize(AllowedValues constraintAnnotation)
{
allowedValues = Arrays.asList(constraintAnnotation.values());
}
@Override
public boolean isValid(List<String> values, ConstraintValidatorContext context) {
return values != null && values.stream()
.allMatch(allowedValues::contains);
}
}
The initialize() method retrieves the allowed values specified in the @AllowedValues annotation and stores them in a list and the isValid() method returns true if all elements in the values list are in the allowedValues list. It also handles null input gracefully by returning false.
The final step is to apply our custom annotation to a List<String> representing various pre-defined departments:
@NotEmpty(message = "Departments list cannot be empty")
@AllowedValues(values = {"Management", "Software Development", "DevOps", "Architect"}, message = "Invalid department provided")
private List<String> department;
The @NotEmpty annotation ensures that the list isn’t empty. Our custom annotation @AllowedValues specifies valid roles like Management, Software Development, DevOps, and Architect and if the validation fails, it returns a custom error message stating Invalid role provided.
5. Conclusion
In this article, we learned to validate lists in Spring, including scenarios where only specific values are allowed. Using built-in annotations like @Pattern and custom annotations, we can ensure our application’s data integrity with minimal effort. The flexibility of @Pattern also makes it useful for validating individual fields in various contexts.
As always, the code presented in this article is available over on GitHub.
The post Validate List of Values in Spring first appeared on Baeldung.