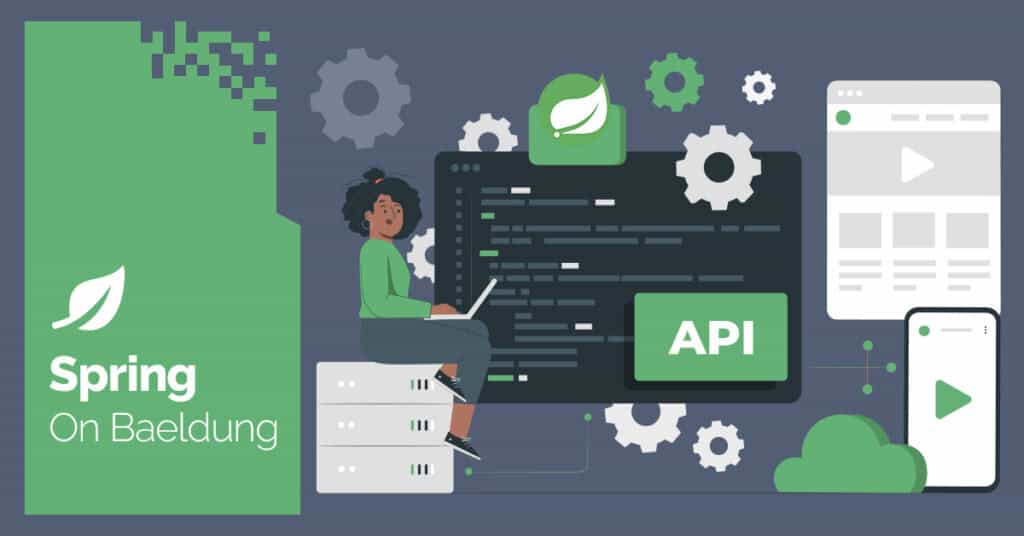
1. Introduction
In this tutorial, we’ll explore SSL, its importance in secure communication, and how the Spring Framework’s unified SSL support simplifies configurations across modules like Spring Boot, Spring Security, and Spring Web.
SSL (Secure Sockets Layer) is a standard security protocol that establishes encrypted links between a web server and a browser, ensuring that data transmitted remains private and secure. It is essential for protecting sensitive information and building trust in web applications.
2. What’s New in Unified SSL Support?
Spring Boot 3.1 introduces the SslBundle, a centralized component for defining and managing SSL configurations. The SslBundle consolidates SSL-related settings, including keystores, truststores, certificates, and private keys, into reusable bundles that can be easily applied to various Spring components.
Key highlights include:
- Centralized Configuration: SSL properties are now managed under the spring.ssl.bundle prefix, providing a single source of truth for SSL settings
- Simplified Management: The framework provides clear defaults, better documentation, and extended support for handling complex use cases like mutual SSL authentication or fine-tuning cipher suites
- Improved Security Practices: Out-of-the-box features ensure adherence to modern security standards, like configurations, support Java KeyStore (JKS), PKCS12, and PEM-encoded certificates, and provide easy configurations for HTTPS enforcement
- Reloading Capabilities: SSL bundles can automatically reload when key material changes, ensuring minimal downtime during updates
The unified SSL support is compatible with various Spring modules, including web servers (Tomcat, Netty), REST clients, and data access technologies. This ensures a consistent SSL experience across the entire Spring ecosystem.
3. Setting Up SSL in Spring Boot
Setting up SSL in Spring Boot is straightforward and has unified support.
3.1. Enabling SSL Using Properties
To enable SSL, we first need to configure the application.properties or application.yml:
server:
ssl:
enabled: true
key-store: classpath:keystore.p12
key-store-password: password
key-store-type: PKCS12
This enables SSL and specifies the location, password, and type of the keystore containing our SSL certificate.
3.2. Configuring Keystore and Truststore Details
To configure a keystone, which holds our server’s certificate and private key, we can use the following properties:
spring:
ssl:
bundle:
jks:
mybundle:
keystore:
location: classpath:application.p12
password: secret
type: PKCS12
For a truststore, which contains certificates for trusted servers, we need to add the following properties:
spring:
ssl:
bundle:
jks:
mybundle:
truststore:
location: classpath:application.p12
password: secret
3.3. Setting Up Self-Signed and CA-Signed Certificates
A self-signed certificate is useful for testing or internal purposes. We can generate one using the keytool command:
$ keytool -genkeypair -alias myalias -keyalg RSA -keystore keystore.p12 -storetype PKCS12 -storepass password
For production environments, it’s recommended to use a CA-signed certificate. To ensure enhanced security and trustworthiness, we can obtain this from a Certificate Authority (CA) and add it to our keystore or truststore.
4. Spring Security and SSL
Spring Security integrates seamlessly with unified SSL support, ensuring secure communication and authentication.
Unified SSL simplifies secure authentication by integrating seamlessly with Spring Security. Developers can use SslBundles to establish secure connections for client and server interactions. We can further enforce HTTPS in our application.
Let’s use the following configuration to enforce HTTPS:
@Configuration
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http.requiresChannel()
.anyRequest()
.requiresSecure();
}
}
Then, to enhance security, we should enable HTTP Strict Transport Security (HSTS):
http.headers()
.httpStrictTransportSecurity()
.includeSubDomains(true)
.maxAgeInSeconds(31536000); // 1 year
This policy ensures browsers only communicate with our application over HTTPS.
5. Customizing SSL Configurations
Fine-tuning SSL settings allows developers to enhance security, optimize performance, and address unique application requirements.
5.1. Fine-Tuning SSL Protocols and Cipher Suites
Spring allows us to customize supported SSL protocols and cipher suites for enhanced security:
server:
ssl:
enabled-protocols: TLSv1.3,TLSv1.2
ciphers: TLS_ECDHE_RSA_WITH_AES_256_GCM_SHA384,TLS_ECDHE_RSA_WITH_AES_128_GCM_SHA256
5.2. Using Programmatic Configuration for Advanced Use Cases
For advanced scenarios, programmatic configurations may be necessary:
HttpServer server = HttpServer.create()
.secure(sslContextSpec -> sslContextSpec.sslContext(sslContext));
This approach provides granular control over SSL settings, especially for dynamic environments.
5.3. Handling Specific Scenarios
We can handle specific scenarios as well by using unified SSL support. For example, enabling mutual SSL authentication:
server:
ssl:
client-auth: need
This setting ensures the server requires a valid client certificate during SSL handshake.
6. Conclusion
In this article, we explored Spring Boot 3.1’s unified SSL support, which makes it easy to configure SSL across Spring applications. The new SslBundle centralizes SSL settings, allowing developers to manage certificates, keystores, and truststores in one place. It simplifies secure communication, integrates seamlessly with Spring Security, and helps enforce HTTPS.
The configuration process is user-friendly, with clear options for enabling SSL, setting up keystores, and customizing security features. Developers can easily fine-tune SSL protocols and handle advanced scenarios like mutual authentication.
The code presented in this article is available over on GitHub.
The post Unified SSL Support in Spring Framework first appeared on Baeldung.