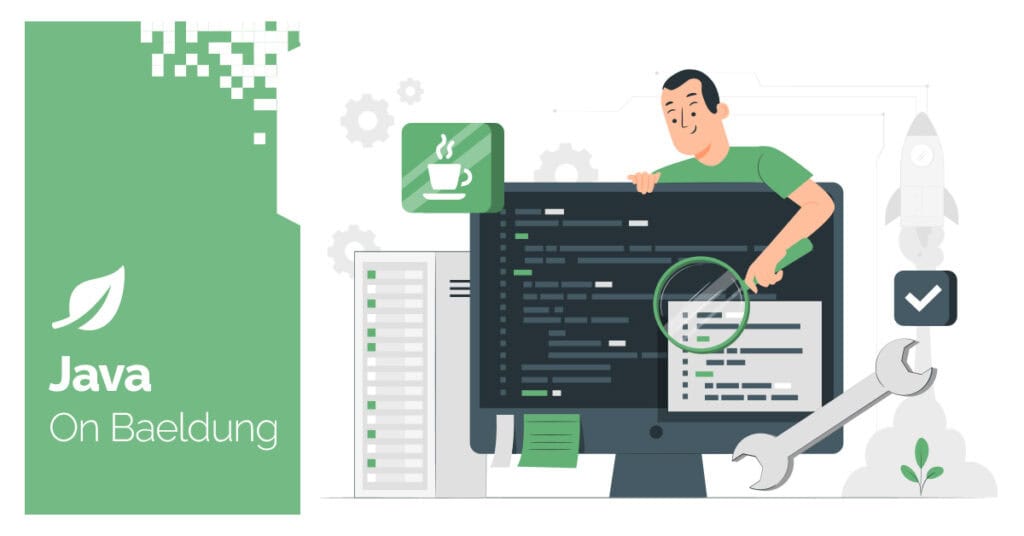
1. Introduction
In this tutorial, we’ll look at a simple example of selecting a date with a date picker control using Selenium WebDriver with Java.
For this test, we’ll use JUnit and Selenium to open the page https://demoqa.com/automation-practice-form and select “2 Dec 2024” using the date picker control for the “Date of Birth” field.
2. Dependencies
First, we need to add the selenium-java and webdrivermanager dependencies to our pom.xml file:
<dependency>
<groupId>org.seleniumhq.selenium</groupId>
<artifactId>selenium-java</artifactId>
<version>4.18.1</version>
</dependency>
<dependency>
<groupId>io.github.bonigarcia</groupId>
<artifactId>webdrivermanager</artifactId>
<version>5.7.0</version>
</dependency>
These allow us to run Java code that invokes the browser and performs actions. In addition, we need JUnit since we’ll be creating a few test cases:
<dependency>
<groupId>org.junit.jupiter</groupId>
<artifactId>junit-jupiter-engine</artifactId>
<version>5.9.2</version>
<scope>test</scope>
</dependency>
We’re ready to create some tests with these dependencies added to our project.
3. Configuration
Next, we need to configure WebDriver. We’ll use Chrome, and we’ll start by downloading its latest version:
@BeforeEach
public void setUp() {
WebDriverManager.chromedriver().setup();
driver = new ChromeDriver();
}
We’re using a method annotated with @BeforeEach to do the initial setup before each test. Next, we use WebDriverManager to get the Chrome driver without explicitly downloading and installing it.
When the test finishes, we’ll close the browser window. We’ll call driver.close() in an @AfterEach method ensuring it’ll be executed even if the test fails:
@AfterEach
public void cleanUp() {
driver.close();
}
4. Find the Date Picker Elements
Now that the basic configuration is done, we’re ready to start the date picker tests. There are several ways to help Selenium pick an element, such as using an ID, CSS selector, or Xpath. However, the date picker can be different from the regular input elements.
4.1. Understanding the Date Picker
A date picker is frequently more complex than other input elements. While there is an input type for dates, many websites don’t use the standard input type.
The reason is that, unlike other input types, the date picker can have different aesthetics, and often, the implementations involve specialized HTML, CSS, and JavaScript code behind the scenes to customize the date picker, such as adding brand colors.
Once the main date control element is clicked, a further set of controls is revealed that offer the opportunity to select a particular year, month, and date. Accordingly, we need to identify the XPath for the year, month, and date input elements.
Let’s start by declaring the website we’ll visit:
private static final String URL = "https://demoqa.com/automation-practice-form";
4.2. Find the Date Control
First, our interaction with a date picker control involves selecting the control to open the date picker. This is usually a button or an input element. For our example, it’s an input element of type text.
The Xpath expression here looks for an input element with the id attribute having the value dateOfBirthInput:
private static final String INPUT_XPATH = "//input[@id='dateOfBirthInput']";
private static final String INPUT_TYPE = "text";
Before we begin writing the actual test, let’s create a simple test to confirm the availability of the date picker control:
@Test
public void givenDemoQAPage_whenFoundDateInput_thenHasAttributeType() {
driver.get(URL);
WebElement inputElement = driver.findElement(By.xpath(INPUT_XPATH));
assertEquals(INPUT_TYPE, inputElement.getAttribute("type"));
}
5. Select a Specific Date
So far, we’ve added a test to identify and confirm that the date picker exists. Next, we want to select a particular date. That involves multiple interactions, which may differ depending on the actual date picker element.
Let’s write a test that picks the date of 2 Dec 2024, and then performs an assertion to check the correct date was picked. This test will involve four steps:
- Click Date Picker Input element
- Select year 2024
- Select month December
- Select day value 2
5.1. Click Input Element
First, let’s click the input element that represents the date control:
driver.get(URL);
WebElement inputElement = driver.findElement(By.xpath(INPUT_XPATH));
inputElement.click();
5.2. Select Year
Next, we’ll define the XPath for the year. This Xpath looks for a div element with the class attribute value react-datepicker__header. This div contains the date picker UI. Within that, we’re interested in a select element with the class attribute of react-datepicker__year-select:
private static final String INPUT_YEAR_XPATH = "//div[@class='react-datepicker__header']"
+ "//select[@class='react-datepicker__year-select']";
Then, we add an explicit wait to allow the JavaScript to run after the click and reveal the Datepicker:
Wait<WebDriver> wait = new FluentWait(driver);
WebElement yearElement = driver.findElement(By.xpath(INPUT_YEAR_XPATH));
wait.until(d -> yearElement.isDisplayed());
After waiting for the actual date picker controls to be revealed, we select the year 2024 from the drop-down:
// Select Year
Select selectYear = new Select(yearElement);
selectYear.selectByVisibleText("2024");
5.3. Select Month
Next, we need to choose the month. So, let’s create the corresponding Xpath. This time, our Xpath looks for a select element with the class attribute having the value react-datepicker__month-select:
private static final String INPUT_MONTH_XPATH = "//div[@class='react-datepicker__header']"
+ "//select[@class='react-datepicker__month-select']";
Now, we can use this to select the month picker:
WebElement monthElement = driver.findElement(By.xpath(INPUT_MONTH_XPATH));
wait.until(d -> monthElement.isDisplayed());
Select selectMonth = new Select(monthElement);
Lastly, we choose the actual month — in this case, December:
// Select Month
selectMonth.selectByVisibleText("December");
We’ve used explicit waits here to ensure that any JavaScript that runs on changes to the date picker runs before we perform the next click.
5.4. Select Day
Finally, we’re ready to pick the particular day of interest. In this case, we’re looking for the second day of December. It’s important to note here that there may be multiple values of the same day in the date picker user interface.
Usually, date picker interfaces also show the last few days from the preceding month or the first few days of the next month. Therefore, the code required to select a particular day may become more complex. Let’s write the XPath expression:
private static final String INPUT_DAY_XPATH = "//div[contains(@class,\"react-datepicker__day\") and "
+ "contains(@aria-label,\"December\") and text()=\"2\"]";
In this expression, we’re using the presence of the class “react-datepicker__day” to select all div elements that represent days of the month. Then, we add additional “and” clauses in the selector to check that the aria-label is December, and finally, that the text value is 2. This ensures that we get exactly one matching element.
Now, we’re ready to select the date:
// Select Day
WebElement dayElement = driver.findElement(By.xpath(INPUT_DAY_XPATH));
wait.until(d -> dayElement.isDisplayed());
dayElement.click();
Let’s finish our test case with an assertion to check that we’ve picked the correct date:
// Check selected date value
assertEquals("02 Dec 2024", inputElement.getAttribute("value"), "Wrong Date Selected");
6. Conclusion
In this article, we’ve learned how to pick a date value with a date picker element using Selenium. Note that the date picker element may not be a standardized input element and that the approach to picking the value may be complex and bespoke.
The general flow uses multiple selectors to identify the date picker input, and then select the year, month, and day in that order. Typically, we expect that after the selection of the day, the full selected date value is available in the date input element and can be used for further code logic or tests.
As always, the source for the article is available over on GitHub.
The post How to Select Date From Datepicker in Selenium first appeared on Baeldung.