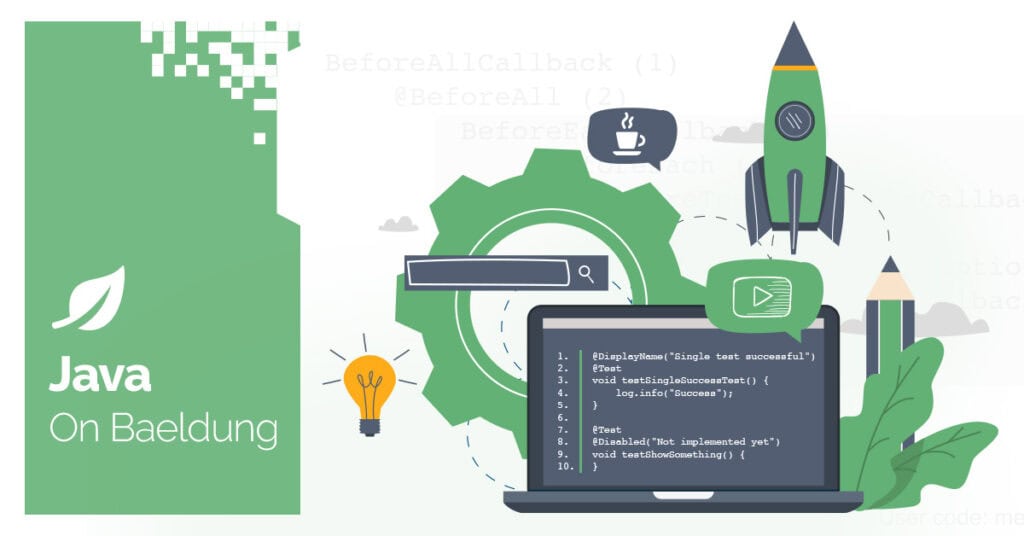
1. Overview
ActiveJ is a lightweight Java framework for high-performance applications. We can use it to create minimalistic and modular applications with a fast startup and a small memory footprint. It provides features like asynchronous I/O, dependency injection, efficient serialization, and reactive programming support.
In this tutorial, we’ll discuss ActiveJ’s main features, including its Inspect module, powerful event loop, and advanced networking capabilities.
2. Inject
We’ll start with ActiveJ Inject. It’s a lightweight and performance-optimized dependency injection library that we can use to set up dependencies between our beans. Let’s see how to use it.
2.1. Dependencies
Let’s add the Active Inject dependencies to our project:
<dependency>
<groupId>io.activej</groupId>
<artifactId>activej-inject</artifactId>
<version>6.0-rc2</version>
</dependency>
2.2. Dependency Injection Using Modules
Let’s create a repository bean:
public class PersonRepository {
private final DataSource dataSource;
public PersonRepository(DataSource dataSource) {
this.dataSource = dataSource;
}
}
In our PersonRepository we just specified the dependency to a DataSource instance. Now let’s create a service class:
public class PersonService {
private final PersonRepository personRepository;
public PersonService(PersonRepository personRepository) {
this.personRepository = personRepository;
}
}
In the PersonService class, we specified the dependency to our PersonRepository.
Next, let’s create a PersonModule class where we’ll configure all the relations between our beans:
public class PersonModule extends AbstractModule {
@Provides
PersonService personService(PersonRepository personRepository) {
return new PersonService(personRepository);
}
@Provides
PersonRepository personRepository(DataSource dataSource) {
return new PersonRepository(dataSource);
}
@Provides
DataSource dataSource() {
return new DataSource() {
//DataSource methods
};
}
}
We extended the AbstractModule class. Additionally, we provided the beans of our PersonService, PersonRepository, and DataSource which have relations between them.
Let’s test the dependency injection behavior:
public class ActiveJTest {
@Test
void givenPersonModule_whenGetTheServiceBean_thenAllTheDependenciesShouldBePresent() {
PersonModule personModule = new PersonModule();
PersonService personService = Injector.of(personModule).getInstance(PersonService.class);
assertNotNull(personService);
PersonRepository personRepository = personService.getPersonRepository();
assertNotNull(personRepository);
DataSource dataSource = personRepository.getDataSource();
assertNotNull(dataSource);
}
}
We’ve created an instance of the PersonModule class. Then, using Injector we obtained the PersonService instance. As we can see, all the dependencies are populated.
3. Async I/O
ActiveJ Async I/O provides components for efficiently writing asynchronous flows. We’ll look at key elements like Promises and the Event Loop to understand this feature better.
3.1. Dependencies
Let’s add the activej-promise dependency:
<dependency>
<groupId>io.activej</groupId>
<artifactId>activej-promise</artifactId>
<version>6.0-rc2</version>
</dependency>
3.2. Promises
Let’s create the model we’ll use:
public record Person(String name, String description) {
}
Now, let’s add some logic to our PersonRepository class:
public Promise<Person> findPerson(String name) {
return Promises
.delay(Duration.ofMillis(100), new Person(name, name + " description"));
}
We’ve added the findPerson() method, which emulates the searching by name process. We used the Promises.delay() to produce an instance of the Person class with some delay. The result will be wrapped with Promise instance.
Next, let’s create another model that we’ll produce in the service layer:
public record VerifiedPerson(String name, String description, String notes, String result) {
}
In VerifiedPerson we have a person’s information and a verification result.
Let’s add the service business logic in the PersonService class:
private Promise<String> findPersonNotes(String name) {
return Promise.of(name + " notes");
}
In the findPersonNotes() method, we emulate the person notes obtaining process. The result is also wrapped by Promise using the Promise.of() method.
Next, let’s add the verification method:
private VerifiedPerson verify(VerifiedPerson person) {
if(person.description().startsWith("Good")) {
return new VerifiedPerson(person.name(), person.description(), person.notes(), "SUCCESS");
}
return new VerifiedPerson(person.name(), person.description(), person.notes(), "FAIL");
}
Here we emulated the verification process adding the verification result based on the person’s description.
Now we finalize our flow and combine all the operations:
public Promise<VerifiedPerson> findAndVerifyPerson(String name) {
return personRepository.findPerson(name)
.combine(findPersonNotes(name),
(person, notes) -> new VerifiedPerson(person.name(), person.description(), notes, null))
.map(person -> verify(person));
}
In our final method, we combine promises from the repository findPerson() and service findPersonNotes() methods using the combine() method of the Promise class. Then we verify the prepared model and return the Promise with the final result.
3.3. Event Loop
The ActiveJ Eventloop executes code asynchronously within event loops and threads. Let’s see how we can use it to run our Promise-based flow:
@Test
void givenEventloop_whenCallFindAndVerifyPerson_thenExpectedVerificationResultShouldBePresent() {
PersonModule personModule = new PersonModule();
PersonService personService = Injector.of(personModule).getInstance(PersonService.class);
Eventloop eventloop = Eventloop.create();
eventloop.run();
personService.findAndVerifyPerson("Good person")
.whenResult(verifiedPerson -> assertEquals("SUCCESS", verifiedPerson.result()));
}
We obtained the PersonService from the PersonModule. Then we created an instance of Eventloop using the Eventloop.create() method and started it using the run() method. Next, we called the findAndVerifyPerson() method. If we miss the run() method, at this point we face the exception:
IllegalStateException: No reactor in current thread
4. HTTP Server
Another great ActiveJ feature we’re going to see is the high-performance asynchronous HTTP server.
4.1. Dependencies
Let’s start by adding the ActiveJ HTTP dependency:
<dependency>
<groupId>io.activej</groupId>
<artifactId>activej-http</artifactId>
<version>6.0-rc2</version>
</dependency>
Next, let’s add the Jackson Databind dependency:
<dependency>
<groupId>com.fasterxml.jackson.core</groupId>
<artifactId>jackson-databind</artifactId>
<version>2.17.0</version>
</dependency>
4.2. REST Endpoint Example
Now, let’s expose our VerifiedPerson flow through an HTTP endpoint. We start from the PersonController class:
public class PersonController implements AsyncServlet {
private final PersonService personService;
private final ObjectMapper objectMapper;
public PersonController(PersonService personService) {
this.personService = personService;
this.objectMapper = new ObjectMapper();
}
@Override
public Promise<HttpResponse> serve(HttpRequest httpRequest) {
return personService.findAndVerifyPerson(httpRequest.getQueryParameter("name"))
.map((p) -> HttpResponse.ok200().withJson(objectMapper.writeValueAsString(p)).build())
.mapException(e -> e);
}
}
Here we’ve implemented the AsyncServlet interface and overridden the serve() method. Inside this method, we get the result of the PersonService findAndVerifyPerson() method. Then we map it into the JSON and return it as an HTTP response body with the 200 response code. The exceptional case is mapped separately and by default returns a 500 status code.
Let’s add this controller to the injection context:
public class PersonModule extends AbstractModule {
@Provides
PersonController personController(PersonService personService) {
return new PersonController(personService);
}
//Other beans
}
We’ve added the PersonController into a PersonModule and configured its dependencies.
Finally, let’s introduce our HTTP server code:
public class ActiveJIntegrationTest {
private static final ObjectMapper objectMapper = new ObjectMapper();
private static HttpServer server;
private static HttpClient client;
private static int port;
private static Eventloop eventloop;
@BeforeAll
static void setUp() throws Exception {
eventloop = Eventloop.create();
PersonModule personModule = new PersonModule();
PersonController personController = Injector.of(personModule).getInstance(PersonController.class);
RoutingServlet servlet = RoutingServlet.builder(eventloop)
.with(HttpMethod.GET,"/person", personController)
.build();
server = HttpServer.builder(eventloop, servlet)
.withListenPort(8080)
.build();
server.listen();
port = server.getListenAddresses().get(0).getPort();
InetAddress dnsServerAddress = InetAddress.getByName("8.8.8.8");
DnsClient dnsClient = DnsClient.builder(eventloop, dnsServerAddress).build();
client = HttpClient.builder(eventloop, dnsClient).build();
}
@AfterAll
static void tearDown() {
if (server != null) {
server.close();
}
}
}
We’ve set up the HttpServer instance. Then we added the HTTP mapping using the RoutingServlet. Finally, we started the server using the listen() method and prepared an asynchronous HTTP client.
Now let’s call our endpoint and check the result:
@Test
void givenHttpServer_whenCallPersonEndpoint_thenExpectedVerificationResultShouldPresentInResponse() {
HttpRequest request = HttpRequest.get("http://localhost:" + port + "/person?name=my-name").build();
client.request(request)
.whenResult(response -> {
assertEquals(response.getCode(), 200);
response.loadBody()
.whenResult(body -> {
try {
VerifiedPerson responseData = objectMapper.readValue(body.getArray(),
VerifiedPerson.class);
assertEquals(responseData.result(), "FAIL");
eventloop.breakEventloop();
} catch (Exception e) {
throw new RuntimeException(e);
}
});
});
eventloop.run();
}
We called our endpoint and asynchronously obtained the expected result. Since we want to stop the server after the test, we called the breakEventloop() method of the Eventloop to stop the execution.
5. Conclusion
In this article, we’ve learned about the key features of the ActiveJ framework. With them, we can already build efficient and lightweight web applications. However, this framework offers much more. We can use it for data processing, distributed systems, and many other cases. Its modular nature helps us avoid overloaded projects and include only the necessary components.
As always, the code is available over on GitHub.
The post Introduction to ActiveJ first appeared on Baeldung.