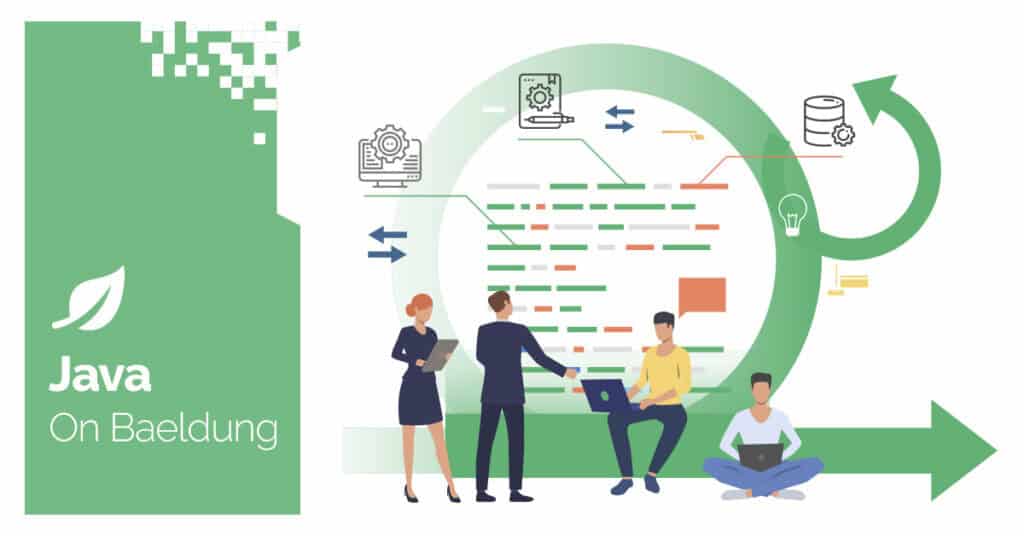
1. Overview
Managing numeric data effectively is a key aspect of Java programming, as selecting the appropriate data type can greatly influence performance and accuracy. The float and double data types are two widely used options for handling decimal numbers. Although they share a common purpose, they vary significantly regarding precision, memory requirements, and typical applications.
This article explores these differences in detail, aiming to guide developers in choosing the right type for tasks such as scientific computations, graphics processing, and financial analyses.
2. Key Characteristics and Differences
Java provides two primitive data types for floating-point arithmetic: float and double. Both adhere to the IEEE 754 standard, ensuring consistent behavior across platforms. However, their size, precision, and performance differ significantly.
2.1. Memory Size
The memory size of float and double is a fundamental distinction that directly impacts their storage capabilities and memory consumption.
A float is a 32-bit single-precision floating-point type, occupying four bytes of memory. This compact size makes it well-suited for memory-constrained environments like embedded systems and mobile devices. Additionally, its smaller footprint can help minimize cache misses and boost performance in memory-intensive applications.
In contrast, a double is a 64-bit double-precision floating-point type, requiring eight bytes of memory. While it demands more storage, this larger size allows it to represent values with higher precision and a broader range, making it indispensable for tasks involving complex calculations or large datasets.
To illustrate this difference in memory usage, we can use the following test case:
@Test
public void givenMemorySize_whenComparingFloatAndDouble_thenDoubleRequiresMoreMemory() {
assertEquals(4, Float.BYTES, "Float should occupy 4 bytes of memory");
assertEquals(8, Double.BYTES, "Double should occupy 8 bytes of memory");
}
This test confirms that float uses 4 bytes, while double uses 8 bytes, highlighting the difference in memory requirements between these two data types.
2.2. Precision
Precision defines the number of significant digits a data type can represent accurately, and the difference between float and double has notable implications for their usage.
A float can handle up to seven significant decimal digits, meaning values exceeding this precision may be rounded or truncated, potentially introducing inaccuracies in calculations with high precision requirements.
On the other hand, a double supports up to 15 significant decimal digits, making it the preferred choice for applications such as scientific computations and financial modeling, where precision is critical. The greater precision of double helps minimize rounding errors, ensuring more dependable results.
To illustrate this difference, let’s consider the following example:
@Test
public void givenPrecisionLimits_whenExceedingPrecision_thenFloatTruncatesAndDoubleMaintains() {
float floatValue = 1.123456789f;
assertEquals(1.1234568f, floatValue, "Float should truncate beyond 7 digits");
double doubleValue = 1.1234567891234566d; // Exceeds 15 digits of precision for double
assertEquals(1.123456789123457, doubleValue, 1e-15, "Double should round beyond 15 digits");
}
This test demonstrates that values exceeding the precision limit of float are truncated, while double maintains accuracy up to 15 digits and rounds beyond that. The delta of 1e-15 accounts for minor rounding differences in double precision.
2.3. Range
The range of a floating-point data type defines the smallest and largest values it can represent, and the ranges of float and double differ significantly.
A float offers a range of approximately -3.4e-38 to +3.4e+38, which is sufficient for many standard applications. However, this range can fall short in scenarios involving extremely large or small numbers.
In contrast, a double extends this range significantly, from -1.7e-308 to +1.7e+308, making it the preferred choice for applications that demand greater numerical reach, such as scientific simulations or astronomical calculations.
To demonstrate, let’s consider the following test case:
public void givenRangeLimits_whenExceedingBounds_thenFloatUnderflowsAndDoubleHandles() {
float largeFloat = 3.4e38f;
assertTrue(largeFloat > 0, "Float should handle large positive values");
float smallFloat = 1.4e-45f;
assertTrue(smallFloat > 0, "Float should handle very small positive values");
double largeDouble = 1.7e308;
assertTrue(largeDouble > 0, "Double should handle extremely large values");
double smallDouble = 4.9e-324;
assertTrue(smallDouble > 0, "Double should handle extremely small positive values");
}
This test highlights how float and double behave near their respective range limits. While float may experience limitations or even underflow to zero for very small values, double accurately represents numbers across a much broader spectrum.
2.4. Performance
Performance considerations for float and double often revolve around their size and precision. float might be slightly faster on some older hardware due to its smaller size, allowing for quicker processing and reduced memory bandwidth usage. This makes it suitable for performance-critical applications where precision is less important.
On modern hardware, the performance difference between float and double is negligible. Most processors are optimized for 64-bit operations, meaning double calculations may execute as quickly as the float.
Therefore, the choice should focus on the precision and range requirements rather than raw performance.
2.5. Summary
The table below highlights the key characteristics of float and double, concisely referencing their distinctions. It serves as a guide to help developers select the appropriate data type based on factors like memory usage, precision, and common applications:
Feature | float | double |
---|---|---|
Size | 32-bit (4 bytes) | 64-bit (8 bytes) |
Precision | ~7 significant decimal digits | ~15 significant decimal digits |
Range | -3.4e-38 to +3.4e+38 | -1.7e-308 to +1.7e+308 |
Performance | Slightly faster on older hardware | Comparable on modern hardware |
3. Common Pitfalls
When working with float and double, we may encounter several common issues.
Accumulating small rounding errors over multiple calculations can lead to significant inaccuracies, making it essential to consider alternatives like BigDecimal for financial computations where precision is critical. Additionally, using values near the boundaries of float and double may result in overflows or underflows, leading to unexpected results.
For instance, attempting to store a value smaller than the representable float range, such as 1e-50f, can cause an underflow to zero. This is because the smallest normalized float value is approximately 1.4e-45f. Values smaller than this will become denormalized (subnormal) numbers or, if they’re too small to be represented as denormalized values, will underflow to zero.
This behavior is demonstrated in the following test case:
@Test
public void givenUnderflowScenario_whenExceedingFloatRange_thenFloatUnderflowsToZero() {
float underflowValue = 1.4e-45f / 2; // Smaller than the smallest normalized float value
assertEquals(0.0f, underflowValue, "Float should underflow to zero for values smaller than the smallest representable number");
}
This test checks that when a value exceeds the lower bound of the float‘s range, it correctly underflows to zero, as expected for values too small to be represented.
4. Conclusion
Choosing between float and double in Java requires understanding the trade-offs in precision, memory usage, and application needs. While float is ideal for memory-constrained and performance-critical environments, double is preferred for applications requiring higher precision and a broader range of values.
By assessing the application’s requirements, we can ensure both efficient and accurate numeric computations.
As always, the code in the article is available over on GitHub.
The post Float vs. Double in Java first appeared on Baeldung.