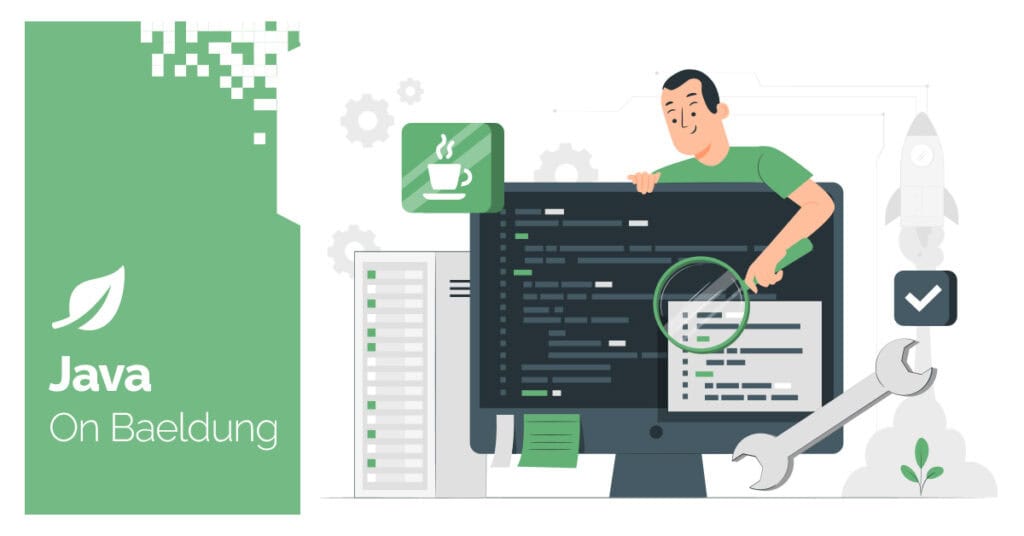
1. Overview
In this short tutorial, we’ll learn how to fix the Hibernate QueryParameterException: “no argument for ordinal parameter”.
First, we’ll understand the root cause behind the exception. Then, we’ll illustrate how to reproduce it, and finally, we’ll learn how to solve it.
2. Understanding the Exception
Before jumping to the solution, let’s take a moment to understand the exception and its stack trace.
Typically, Hibernate throws QueryParameterException to signal an issue with the query parameters. It denotes that a specified parameter is invalid or not found.
Furthermore, the message “no argument for ordinal parameter” indicates that Hibernate fails to find a suitable argument for a defined parameter. A common cause of this exception is neglecting to provide a value for a defined parameter in a Hibernate query.
3. Practical Example
Now that we know what causes Hibernate to fail with QueryParameterException, let’s go down the rabbit hole and see how to reproduce it using a practical example.
First of all, let’s consider the Employee entity class:
@Entity
public class Employee {
@Id
private int id;
private String firstName;
private String lastName;
// standard getters and setters
}
In this example, an employee is defined by their identifier, first name, and last name.
The @Entity annotation is used to specify that the Employee class is a JPA entity, while the @Id annotation marks the field that represents the primary key.
Next, let’s create an HQL query with two parameters and pretend to forget to specify a value for the second one:
@Test
void whenMissingParameter_thenThrowQueryParameterException() {
assertThatThrownBy(() -> {
String selectQuery = """
FROM Employee
WHERE firstName = ?1
AND lastName = ?2
""";
Query<Employee> query = session.createQuery(selectQuery, Employee.class);
query.setParameter(1, "Jean");
query.list();
}).isInstanceOf(QueryParameterException.class)
.hasMessageContaining("No argument for ordinal parameter");
}
As we can see, Hibernate throws the exception because we didn’t use setParameter() to set a value for the lastName parameter.
4. Fixing the Exception
The easiest solution to solve QueryParameterException in our case is to specify a valid value for the second parameter before executing the query. So, let’s add a new test case and use setParameter() to set the last name:
@Test
void whenDefiningAllParameters_thenCorrect() {
String selectQuery = """
FROM Employee
WHERE firstName = ?1
AND lastName = ?2
""";
Query<Employee> query = session.createQuery(selectQuery, Employee.class);
query.setParameter(1, "Jean")
.setParameter(2, "Smith");
assertThat(query.list()).isNotNull();
}
Unsurprisingly, the test case is successfully executed and doesn’t fail with QueryParameterException.
5. Conclusion
In this short article, we explored what causes Hibernate to fail with QueryParameterException: “no argument for ordinal parameter”. Along the way, we demonstrated how to reproduce the exception and how to fix it.
As always, the full source code of the examples is available over on GitHub.
The post Fixing Hibernate QueryParameterException: No Argument for Ordinal Parameter first appeared on Baeldung.