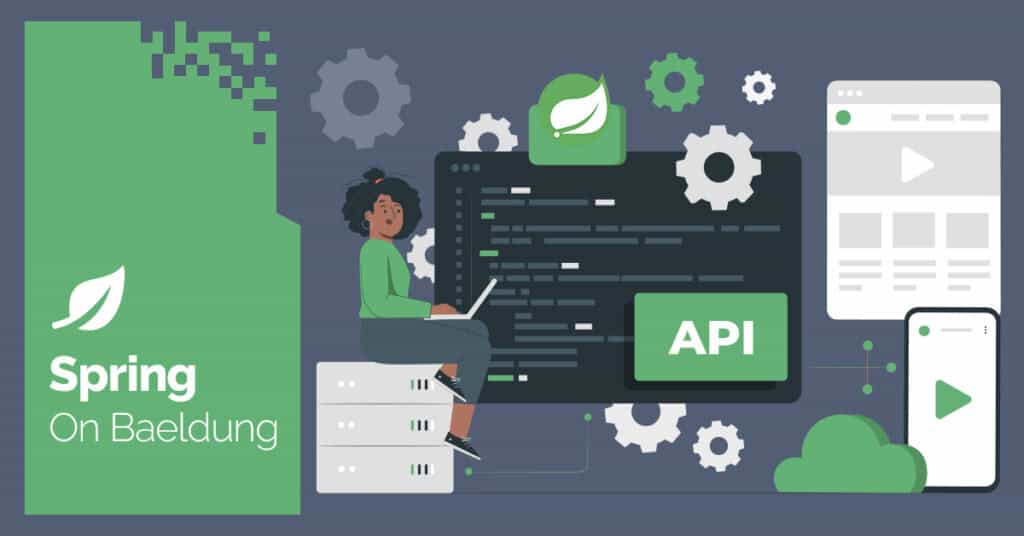
1. Introduction
Spring WebFlux is a reactive programming framework that facilitates asynchronous, non-blocking communication. A key aspect of working with WebFlux is handling Mono objects, representing a single asynchronous result. In real-world applications, we often need to transform one Mono object to another, whether to enrich data, handle external service calls, or restructure the payload.
In this tutorial, we’ll explore how to convert a Mono object into another Mono object using various approaches provided by Project Reactor.
2. Converting Mono Object
Before we explore the various ways to transform Mono objects, let’s set up our coding example. We’ll use book-borrow examples throughout our tutorial to demonstrate different transformation methods. To capture this scenario, we’ll work with three key classes.
User class to represent a library user:
public class User {
private String userId;
private String name;
private String email;
private boolean active;
// standard setters and getters
}
Each user is uniquely identified by a userId and has personal details like name and email. Additionally, there’s an active flag to indicate whether the user is currently eligible to borrow books.
Book class to represent the library’s collection of books:
public class Book {
private String bookId;
private String title;
private double price;
private boolean available;
//standard setters and getters
}
Each book is identified by a bookId and has attributes like title and price. The available flag indicates whether the book can be borrowed.
BookBorrowResponse class to encapsulate the result of a borrowing operation:
public class BookBorrowResponse {
private String userId;
private String bookId;
private String status;
//standard setters and getters
}
This class ties together the userId and bookId involved in the process and provides a status field to indicate whether the borrowing was accepted or rejected.
3. Synchronous Transformations with map()
The map operator applies a synchronous function to the data inside Mono. It suits lightweight operations like formatting, filtering, or simple computations. For example, if we want to get the email address from the Mono user, we can use the map to convert it:
@Test
void givenUserId_whenTransformWithMap_thenGetEmail() {
String userId = "U001";
Mono<User> userMono = Mono.just(new User(userId, "John", "john@example.com"));
Mockito.when(userService.getUser(userId))
.thenReturn(userMono);
Mono<String> userEmail = userService.getUser(userId)
.map(User::getEmail);
StepVerifier.create(userEmail)
.expectNext("john@example.com")
.verifyComplete();
}
4. Asynchronous Transformations with flatMap()
The flatMap() method transforms each emitted item from Mono into another Publisher. It’s especially useful when the transformation requires a new asynchronous process, such as making another API call or querying a database. flatMap() flattens the result into a single sequence when the transformation result is a Mono.
Let’s look into our book borrowing system. When a user requests to borrow a book, the system validates the user’s membership status and then checks if the book is available. If both checks pass, the system processes the borrowing request and returns a BookBorrowResponse:
public Mono<BookBorrowResponse> borrowBook(String userId, String bookId) {
return userService.getUser(userId)
.flatMap(user -> {
if (!user.isActive()) {
return Mono.error(new RuntimeException("User is not an active member"));
}
return bookService.getBook(bookId);
})
.flatMap(book -> {
if (!book.isAvailable()) {
return Mono.error(new RuntimeException("Book is not available"));
}
return Mono.just(new BookBorrowResponse(userId, bookId, "Accepted"));
});
}
In this example, the operations, such as retrieving user and book details, are asynchronous and return Mono objects. Using flatMap(), we can chain these operations in a readable and logical manner, without nesting multiple levels of Mono. Each step in the sequence depends on the result of the previous step. For example, book availability is only checked if the user is active. flatMap() ensures we can make these decisions dynamically while keeping the flow reactive.
5. Reusable Logic with transform() Method
The transform() method is a versatile tool that allows us to encapsulate reusable logic. Instead of repeating transformations across multiple parts of the application, we can define them once and apply them whenever required. This promotes code reusability, separation of concerns, and readability.
Let’s look into an example where we need to return the final price of a book after applying tax and discount:
public Mono<Book> applyDiscount(Mono<Book> bookMono) {
return bookMono.map(book -> {
book.setPrice(book.getPrice() - book.getPrice() * 0.2);
return book;
});
}
public Mono<Book> applyTax(Mono<Book> bookMono) {
return bookMono.map(book -> {
book.setPrice(book.getPrice() + book.getPrice() * 0.1);
return book;
});
}
public Mono<Book> getFinalPricedBook(String bookId) {
return bookService.getBook(bookId)
.transform(this::applyTax)
.transform(this::applyDiscount);
}
In this example applyDiscount() method applies a discount of 20% and the applyTax() method applies a 10% Tax. The transform method applies both methods in the pipeline and returns Mono of Book with the final price.
6. Merging Data from Multiple Sources
The zip() method combines multiple Mono objects and produces a single result. It does not merge results concurrently but waits for all Mono objects to emit before applying the combinator function.
Let’s reiterate our book borrow example, where we fetch user info and book info to create a BookBorrowResponse:
public Mono<BookBorrowResponse> borrowBookZip(String userId, String bookId) {
Mono userMono = userService.getUser(userId)
.switchIfEmpty(Mono.error(new RuntimeException("User not found")));
Mono bookMono = bookService.getBook(bookId)
.switchIfEmpty(Mono.error(new RuntimeException("Book not found")));
return Mono.zip(userMono, bookMono,
(user, book) -> new BookBorrowResponse(userId, bookId, "Accepted"));
}
In this implementation, the zip() method ensures the user and book information is available before creating the response. If the user or book retrieval fails (e.g. if the user doesn’t exist or the book is not available), the error will propagate, and the combined Mono terminates with the appropriate error signal.
7. Conditional Transformations
By combining filter() and switchIfEmpty() methods, we can apply conditional logic to transform a Mono object based on a predicate. If the predicate is true, the original Mono is returned and if it’s false, the Mono switches to a different one provided by switchIfEmpty() or vice versa.
Let’s consider a scenario where we want to apply a discount only if the user is active, else return without discount:
public Mono<Book> conditionalDiscount(String userId, String bookId) {
return userService.getUser(userId)
.filter(User::isActive)
.flatMap(user -> bookService.getBook(bookId).transform(this::applyDiscount))
.switchIfEmpty(bookService.getBook(bookId))
.switchIfEmpty(Mono.error(new RuntimeException("Book not found")));
}
In this example, we fetch a Mono of User using userId. The filter method checks if the user is active. If the user is active, we return a Mono of Book after applying the discount. If the user is inactive, the Mono becomes empty, and the switchIfEmpty() method kicks in to fetch the book without applying a discount. Finally, if the book itself does not exist, another switchIfEmpty() ensures an appropriate error is propagated, making the entire flow robust and intuitive.
8. Error Handling During Transformations
Error handling ensures resilience in transformations, allowing graceful fallback mechanisms or alternative data sources. When a transformation fails, proper error handling helps in recovering gracefully, logging issues, or returning alternative data.
The onErrorResume() method is used to recover from errors by providing an alternative Mono. This is especially useful when we want to provide default data or fetch data from an alternative source.
Let’s revisit our book borrow example; if any error is thrown while fetching either the User or Book object, we handle the failure gracefully by returning a BookBorrowResponse object with “Rejected” status:
public Mono<BookBorrowResponse> handleErrorBookBorrow(String userId, String bookId) {
return borrowBook(userId, bookId)
.onErrorResume(ex -> Mono.just(new BookBorrowResponse(userId, bookId, "Rejected")));
}
This error-handling strategy ensures that even in failure scenarios, the system responds predictably and maintains a seamless user experience.
9. Best Practices for Converting Mono Objects
When converting Mono objects, it is essential to follow some best practices to ensure our reactive pipelines are clean, efficient, and maintainable. When we need simple, synchronous transformations like enriching or modifying data, the map() method is a perfect choice, while flatMap() is ideal for tasks involving asynchronous workflows, such as calling an external API or querying databases. To keep pipelines clean and reusable, we encapsulate logic with the transform() method, promoting modularity and separation of concerns. To maintain readability, we should prefer chaining over nesting operations.
Error handling plays a key role in ensuring resilience. By using methods like onErrorResume(), we can gracefully manage errors by providing fallback responses or alternative data sources. Finally, validating inputs and outputs at every stage helps prevent issues from propagating downstream, ensuring a robust and scalable pipeline.
10. Conclusion
In this tutorial, we’ve learned various ways to convert one Mono object to another. It’s essential to understand the right operator for the job, be it map(), flatMap(), or transform(). With these techniques and applying best practices, we can build a flexible and maintainable reactive pipeline in Spring WebFlux.
As always, all code snippets used in this article are available over on GitHub.
The post Convert Mono Object to Another Mono Object in Spring WebFlux first appeared on Baeldung.