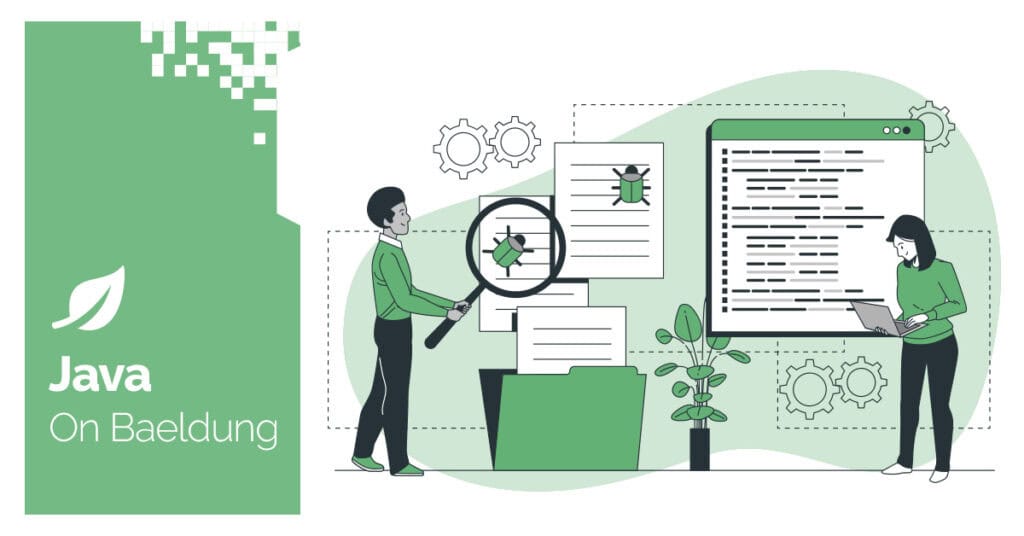
1. Introduction
In this tutorial, we’ll learn how to remove insignificant zeros from a number represented in a String, including leading and trailing zeros. We’ll explore several ways to achieve this, including using the standard core Java packages.
We’ll take examples of positive and negative number Strings with each implementation.
2. Using String.replaceAll()
First, let’s take a look at the String replaceAll() method. The replaceAll() method uses a regex pattern to replace the content. We’ll call the replaceAll() method to remove leading and trailing zeros from the String.
We want to match all zeroes at the beginning or end of a String and replace them with an empty String. Firstly, we’ll check if the String contains a dot character; we’ll recursively call the replaceAll() method to remove leading and trailing zeros from the input. Otherwise, we need to remove zeros only from the start of the String.
We’ll take an example of a decimal number for better understanding:
@Test
public void givenPositiveNumberString_whenUsingStringReplaceAll_thenInsignificantZeroRemoved() {
String positiveNumber = "001200.35000";
positiveNumber = positiveNumber.contains(".") ? positiveNumber.replaceAll("^(-?)0+(\\d+)", "$1$2")
.replaceAll("0+$", "")
.replaceAll("\\.$", ".0") : positiveNumber.replaceAll("^(-?)0+(\\d+)", "$1$2");
assertEquals("1200.35", positiveNumber);
}
@Test
public void givenNegativeNumberString_whenUsingStringReplaceAll_thenInsignificantZeroRemoved() {
String negativeNumber = "-0015.052200";
negativeNumber = negativeNumber.contains(".") ? negativeNumber.replaceAll("^(-?)0+(\\d+)", "$1$2")
.replaceAll("0+$", "")
.replaceAll("\\.$", ".0") : negativeNumber.replaceAll("^(-?)0+(\\d+)", "$1$2");
assertEquals("-15.0522", negativeNumber);
}
Here, the first replaceAll() method call will ensure we remove any zeros at the start of the String. If it contains a negative sign, we need to keep that sign along with the digits. The second call will remove trailing zeros from the end of the String. The third call checks if only a dot remains at the end of the String. If so, we’ll replace it with “.0” to ensure a decimal number.
As a result, we’ll get the required String without zeros at the start or end.
3. Using DecimalFormat
The DecimalFormat class allows us to provide a pattern to format a number according to our requirements. The only catch is that it restricts the number of digits after a decimal point based on the given pattern.
Let us use the DecimalFormat to remove the insignificant zeros. We’ll also provide a pattern in the DecimalFormat:
@Test
public void givenPositiveNumberString_whenUsingDecimalFormat_thenInsignificantZeroRemoved() {
String positiveNumber = "001200.35000";
positiveNumber = new DecimalFormat("0.0#####").format(Double.valueOf(positiveNumber));
assertEquals("1200.35", positiveNumber);
}
@Test
public void givenNegativeNumberString_whenUsingDecimalFormat_thenInsignificantZeroRemoved() {
String negativeNumber = "-0015.052200";
negativeNumber = new DecimalFormat("0.0#####").format(Double.valueOf(negativeNumber));
assertEquals("-15.0522", negativeNumber);
}
We created a DecimalFormat instance by providing a pattern, which we’ll use to format the given String. In the pattern, we used “#” meaning that if a digit is other than zero, it will be included in the output or removed and the number of “#” in the format means it will format the number with a maximum of that many decimal places.
As a result, we get the String without zeros at the start or end.
4. Using BigDecimal
The BigDecimal class provides a method to remove the insignificant zeros from the given String. The BigDecimal initialization will, by default, remove leading zeros from the string. BigDecimal provides the stripTrailingZeros() method which removes trailing zeros from the String. After removing trailing zeros from a decimal number, if the fraction part is empty, then we will get the number without a decimal point:
@Test
public void givenPositiveNumberString_whenUsingBigDecimal_thenInsignificantZeroRemoved() {
String positiveNumber = "001200.35000";
positiveNumber = new BigDecimal(positiveNumber).stripTrailingZeros()
.toPlainString();
assertEquals("1200.35", positiveNumber);
}
@Test
public void givenNegativeNumberString_whenUsingBigDecimal_thenInsignificantZeroRemoved() {
String negativeNumber = "-0015.052200";
negativeNumber = new BigDecimal(negativeNumber).stripTrailingZeros()
.toPlainString();
assertEquals("-15.0522", negativeNumber);
}
We created a BigDecimal instance by passing the String of a number in the argument and then calling the stripTrailingZeros() method to remove all trailing zeros. Finally, we called the toPlainString() method, which returns the String with only significant zeros.
5. Conclusion
In this article, We’ve explored different ways to remove insignificant zeros from the String. We also explained the various limitations of each approach. We should choose the approach based on the application’s specific requirement of the number representation.
All of the code in this article is available over on GitHub.
The post Remove Insignificant Zeros From a Number Represented as a String first appeared on Baeldung.