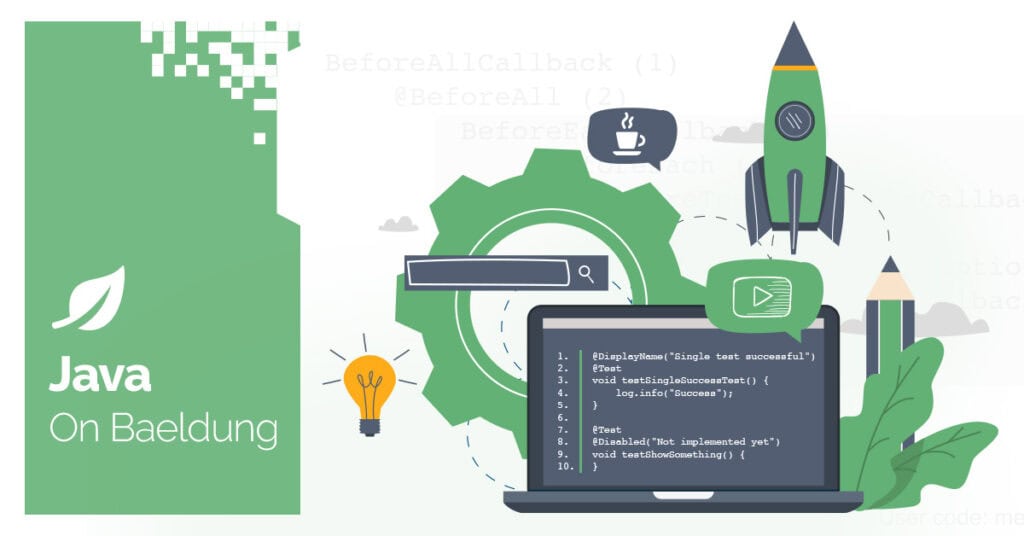
1. Overview
In Java, inheritance is a powerful mechanism for reusing and extending functionalities across classes. By leveraging the super keyword, we can access the methods and properties of a parent class from its subclass.
However, one limitation in Java surprises us: super.super.method() is not allowed.
In this tutorial, we’ll dive into why this is the case and how Java’s design philosophy shapes this behavior.
2. Introduction to the Problem
As usual, let’s understand the problem through examples. Let’s say, we have three classes:
class Person {
String sayHello() {
return "Person: How are you?";
}
}
class Child extends Person {
@Override
String sayHello() {
return "Child: How are you?";
}
String mySuperSayHello() {
return super.sayHello();
}
}
class Grandchild extends Child {
@Override
String sayHello() {
return "Grandchild: How are you?";
}
String childSayHello() {
return super.sayHello();
}
String personSayHello() {
return super.super.sayHello();
}
}
As the code above shows, the three classes have this hierarchy:
[Person] <--subtype-- [Child] <--subtype-- [Grandchild]
Further, all subtypes override the parent class’s sayHello() method.
It’s worth noting that Grandchild has two additional methods: childSayHello() and personSayHello(). Their implementations are straightforward, calling super.sayHello() in childSayHello() and super.super.sayHello() in personSayHello().
However, Java doesn’t compile super.super.sayHello() and complains:
java: <identifier> expected
So next, let’s understand why calling super.super.method() isn’t allowed in Java, and figure out how to achieve calling grandparent class’s methods.
3. Why Calling super.super.method() Is Not Allowed in Java
It’s intentionally designed to prohibit super.super.method() in Java. Next, let’s take figure out why.
3.1. Violating Encapsulation
Java is designed with encapsulation and abstraction as core principles. These principles dictate that a class should only concern itself with its direct parent, not the implementation details of its grandparent or beyond.
Directly calling the grandparent’s method ignores the parent’s role in controlling the inheritance chain, exposing internal logic that the parent might have intentionally hidden or altered.
Next, let’s examine a fresh example that illustrates why Java prohibits using super.super.method() and what issues this could lead to if it were allowed.
3.2. The Players Example
First, let’s declare a Player class:
class Player {
private String name;
private String type;
private int rank;
public Player(String name, String type, int rank) {
this.name = name;
this.type = type;
this.rank = rank;
}
// ...getter and setter methods are omitted
}
The name and rank properties are straightforward. The type property holds which area the player belongs to, such as “football” or “tennis“.
Next, let’s create a set of Player collection types:
class Players {
private List<Player> players = new ArrayList();
protected boolean add(Player player) {
return players.add(player);
}
}
class FootballPlayers extends Players {
@Override
protected boolean add(Player player) {
if (player.getType().equalsIgnoreCase("football")) {
return super.add(player);
}
throw new IllegalArgumentException("Not a football player");
}
}
class TopFootballPlayers extends FootballPlayers {
@Override
protected boolean add(Player player) {
if (player.getRank() < 10) {
return super.add(player);
}
throw new IllegalArgumentException("Not a top player");
}
}
As the code shows, the inheritance hierarchy of the three Player collection classes is as follows:
[Players] <--subtype-- [FootballPlayers] <--subtype--[TopFootballPlayers]
Both FootballPlayers and TopFootballPlayers override the add() method in their superclasses. Of course, they added different checks in the add() method to ensure Player objects only with the required type can be added. For instance, TopFootballPlayers.add() requires the player’s rank to be higher than 10. Then, TopFootballPlayers.add() invokes super.add(), which guarantees only top football players can be added.
A test can quickly show how this inheritance hierarchy works:
Player liam = new Player("Liam", "football", 9);
Player eric = new Player("Eric", "football", 99);
Player kai = new Player("Kai", "tennis", 7);
TopFootballPlayers topFootballPlayers = new TopFootballPlayers();
assertTrue(topFootballPlayers.add(liam));
Exception exEric = assertThrows(IllegalArgumentException.class, () -> topFootballPlayers.add(eric));
assertEquals("Not a top player", exEric.getMessage());
Exception exKai = assertThrows(IllegalArgumentException.class, () -> topFootballPlayers.add(kai));
assertEquals("Not a football player", exKai.getMessage());
As we can see, eric is a football player, but his rank is lower than 10. Therefore, TopFootballPlayers.add() rejects adding it.
Similarly, kai is rejected by the TopFootballPlayers.add() method, too, since he’s a tennis player, although his rank = 7. This is checked using TopFootballPlayers‘ superclass FootballPlayer’s add() method.
3.3. If super.super.add() Were Allowed
Now, let’s say, assuming the super.super.method() call were allowed, we can rewrite TopFootballPlayers.add() in this way:
class TopFootballPlayers extends FootballPlayers {
@Override
protected boolean add(Player player) {
if (player.getRank() < 10) {
return super.super.add(player); // call grandparent's add() method directly
}
throw new IllegalArgumentException("Not a top player");
}
}
Then, we can add any Player instances with the rank < 10. In other words, TopFootballPlayers can add() kai, even if kai is a tennis player. Thus, it breaks the invariant in its parent: FooballPlayers.
3.4. Other Reasons
There are other possible reasons that Java disallows super.super.method().
Java prioritizes simplicity and clarity in its language design. Allowing super.super.method() would introduce unnecessary complexity, requiring the language to dynamically resolve deeper levels of the inheritance hierarchy. This complexity would make the code harder to read and reason about, especially in larger codebases. By restricting access to the immediate parent only, Java ensures that the inheritance chain remains clear and manageable.
Apart from the design simplicity, another reason is that the “super“ may not have a “super”. Thus, super.super doesn’t exist in this case.
We know all classes are subclasses of Object in Java. Therefore, any class we have created has a valid “super“. However, if OurClass inherits directly from Object, ourClass.super is Object, and ourClass.super.super doesn’t exist. This makes super.super invalid.
However, in some situations, we want to call a grandparent class’s method. Next, let’s see how to achieve it.
4. A Workaround: Indirect Call
Java reflection is so powerful that it can do almost anything. Using reflection allows us to achieve super.super.method(), too. But we won’t dive into such reflection implementations in this tutorial, as it still violates encapsulation.
Although super.super.method() is not allowed, Java provides other mechanisms to achieve the desired functionality. For example, we can refactor the parent class: If a subclass needs access to a method from its grandparent, the parent class can expose it explicitly.
Next, let’s show how this is done using our Person-Child-Grandchild:
class Child extends Person {
// ...same code omitted
String mySuperSayHello() {
return super.sayHello();
}
}
class Grandchild extends Child {
// ...same code omitted
String personSayHello() {
return super.mySuperSayHello();
}
}
We aim to call Person.sayHello() from Grandchild. Then, as the code shows, we can expose Person.sayHello() in the Child class Child.mySuperSayHello():
Grandchild aGrandchild = new Grandchild();
assertEquals("Grandchild: How are you?", aGrandchild.sayHello());
assertEquals("Child: How are you?", aGrandchild.childSayHello());
assertEquals("Person: How are you?", aGrandchild.personSayHello());
This approach does the job without violating encapsulation.
5. Conclusion
In this article, we’ve discussed why Java disallows super.super.method(). The absence of super.super.method() isn’t an oversight but a deliberate design choice aligned with encapsulation, abstraction, and simplicity principles.
Java encourages better code organization and maintainability by restricting a subclass to interact only with its immediate parent.
As always, the complete source code for the examples is available over on GitHub.
The post Why super.super.method() is Not Allowed in Java first appeared on Baeldung.