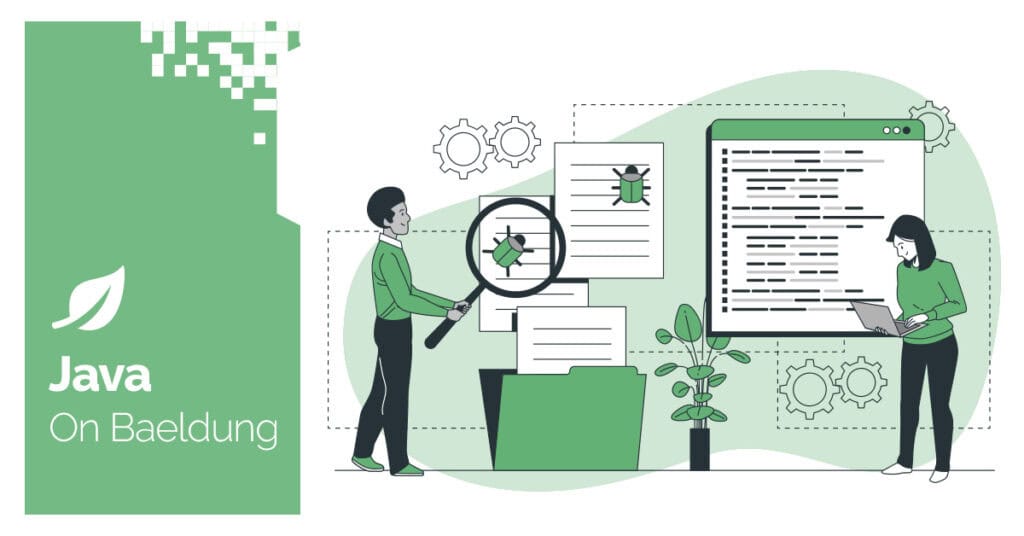
1. Introduction
JUL is Java’s built-in logging framework. It’s simple to use but lacks the flexibility and advanced features offered by modern alternatives. For this reason, many applications choose something else.
But in this world of modular software and third-party dependencies, something in our application may use JUL despite our preference. This makes correctly configuring logs more complex.
In this article, we’ll see how SLF4J‘s JUL-to-SLF4J bridge helps address this issue.
2. Dependencies
To begin, we include the SLF4J dependencies in our project. For Maven projects, we need to add the slf4j-api and jul-to-slf4j dependencies to our pom.xml file:
<dependency>
<groupId>org.slf4j</groupId>
<artifactId>slf4j-api</artifactId>
<version>2.0.16</version>
</dependency>
<dependency>
<groupId>org.slf4j</groupId>
<artifactId>jul-to-slf4j</artifactId>
<version>2.0.16</version>
</dependency>
The jul-to-slf4j artifact consists of a java.util.logging (JUL) handler, namely SLF4JBridgeHandler, which routes all incoming JUL records to the SLF4J API.
Note that an application must also specify the SLF4J API SLF4J logging implementation to use. So, for this article, let’s assume that our app uses slf4j-simple:
<dependency>
<groupId>org.slf4j</groupId>
<artifactId>slf4j-simple</artifactId>
<version>2.0.16</version>
</dependency>
3. Default JUL Logs
Before configuring the bridge, the output will use the default JUL format if a library logs via JUL. For example, consider the following code:
import java.util.logging.Logger;
public class BusinessLogic {
static void julLog(Logger julLogger) {
julLogger.info("This is a JUL info log message!");
julLogger.warning("This is a JUL warning log message!");
}
}
When running this code without the JUL-to-SLF4J bridge, the julLog() produces output similar to:
Jan 19, 2025 11:43:41 PM com.baeldung.BusinessLogic julLog INFO: This is a JUL info log message!
Jan 19, 2025 11:43:41 PM com.baeldung.BusinessLogic julLog WARNING: This is a JUL warning log message!
4. Configuring the Bridge
We can configure the bridge in one of two ways: programmatically or through a logging.properties file. Let’s look at each one.
4.1. Programmatic Configuration
We can programmatically set up the SLF4J bridge in the main application class. This method is straightforward and ensures that the bridge is installed during the initialization phase of our application:
import org.slf4j.bridge.SLF4JBridgeHandler;
import java.util.logging.Logger;
public static void main(String[] args) {
// Remove existing handlers
SLF4JBridgeHandler.removeHandlersForRootLogger();
// Install SLF4J bridge
SLF4JBridgeHandler.install();
// Test output after slf4j bridge was installed
BusinessLogic.julLog(julLogger);
BusinessLogic.slf4jLog(logger);
}
In this code, we first remove any existing JUL handlers to prevent duplicate log entries. Then, we install the SLF4J bridge, which redirects all JUL log records to SLF4J.
4.2. Configuration with logging.properties
Alternatively, we can use JUL’s logging.properties file to configure the bridge declaratively. This method is ideal for applications where modifying the codebase or adding programmatic configuration is not feasible.
To do this, we’ll first create src/main/resources/logging.properties and specify the JUL handler and logging level:
handlers = org.slf4j.bridge.SLF4JBridgeHandler
.level = INFO
In this configuration:
- The handlers line specifies that the SLF4JBridgeHandler should handle logging for the JUL root logger
- The .level property sets the default logging level to INFO, meaning only messages of this level or higher will be logged
JUL uses the logging.properties file in the JAVA_HOME/conf directory by default. To ensure our custom configuration is used, we need to explicitly specify the location of our logging.properties file when running our application. This can be done using a JVM argument:
-Djava.util.logging.config.file=/path/to/logging.properties
This ensures that our custom logging configuration is loaded instead of the default.
Note that this approach works fine, but it has the downside that we need to manage some JUL internals to make it work. The programmatic approach allows us to stick with the SLF4J API for configuration.
5. Test the Logging Configuration
Now that we’ve set up our JUL-to-SLF4J bridge, we can test our logging configuration to ensure everything works as expected.
5.1. Logging via JUL
Even if a third-party library logs using JUL, the log messages will now be routed through SLF4J:
import java.util.logging.Logger;
public class BusinessLogic {
static void julLog(Logger julLogger) {
julLogger.info("This is a JUL info log message!");
julLogger.warning("This is a JUL warning log message!");
}
}
Instead of JUL, these logs will be handled by the SLF4J backend (for example, slf4j-simple) and the julLog() output resembles:
[main] INFO com.baeldung.JULAppWithProgrammaticSLF4J - This is a JUL info log message!
[main] WARN com.baeldung.JULAppWithProgrammaticSLF4J - This is a JUL warning log message!
5.2. Logging Directly via SLF4J
While the previous section showed that we can now use JUL alongside SLF4J, it’s better to declare a SLF4J logger:
public class BusinessLogic {
static void slf4jLog(org.slf4j.Logger logger) {
logger.info("This is an SLF4J info log message!");
logger.error("This is an SLF4J error log message!");
}
}
By testing both JUL-based and SLF4J-based logging, we can confirm that the integration is working correctly.
6. Conclusion
The JUL-to-SLF4J bridge enhances our logging capabilities while maintaining compatibility with JUL. By integrating SLF4J, we gain a powerful logging API and improved flexibility. This bridge is a valuable addition for developers aiming to improve logging practices and ensure maintainability. As usual, the code can be found over on GitHub.
The post JUL to SLF4J Bridge first appeared on Baeldung.