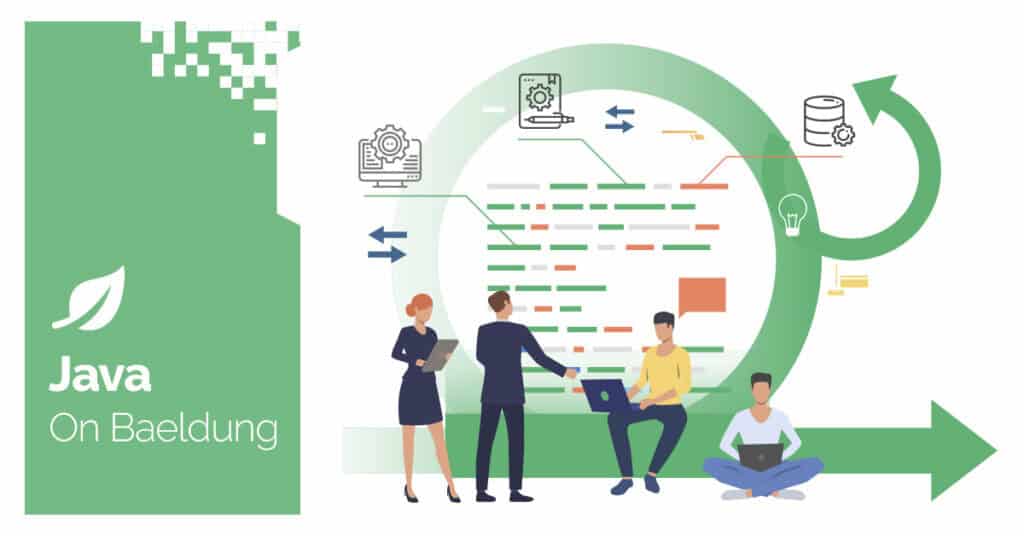
1. Introduction
In this tutorial, we’ll explore fixing the exception: Cannot issue data manipulation statements with executeQuery().
It’s not common to encounter this problem when working with JDBC to interact with databases, but fortunately, it’s easily solved.
2. Understand the Exception
The error message itself tells us where the error might be, but let’s get into the depth of the problem.
2.1. What Does It Mean?
The error Cannot issue data manipulation statements with executeQuery() occurs when our code attempts to execute an INSERT, UPDATE, or DELETE statement using the executeQuery() method.
The method executeQuery() from Statement or PreparedStatement objects is specifically designed to handle SELECT queries. If we inspect the method signature, we’ll notice that it returns the ResultSet instance, which contains the rows retrieved from the database.
This exception occurs when using Connector/J to connect to MySQL, but other databases enforce the same rule. In this situation, they throw a similar error with a different error message.
It’s worth noting that in newer versions of MySQL Connector/J, this error message has been slightly updated. It now reads as:
java.sql.SQLException: Statement.executeQuery() cannot issue statements that do not produce result sets.
2.2. Common Scenarios That Trigger the Exception
Let’s look at a code example to understand better what triggers the exception. As mentioned previously, we’ll use a MySQL database.
As a first step, we create a simple table for our example:
CREATE TABLE IF NOT EXISTS users (
id INT PRIMARY KEY AUTO_INCREMENT,
username VARCHAR(50),
email VARCHAR(50)
)
We can now try to perform a query that isn’t a SELECT statement. Let’s go with a simple INSERT statement inside a test and confirm that an exception is thrown:
@Test
void givenInsertSql_whenExecuteQuery_thenAssertSqlExceptionThrown() throws SQLException {
String insertSql = "INSERT INTO users (username, email) VALUES (?, ?)";
PreparedStatement insertStatement = connection.prepareStatement(insertSql);
insertStatement.setString(1, USERNAME);
insertStatement.setString(2, EMAIl);
SQLException exception = assertThrows(SQLException.class, insertStatement::executeQuery);
assertEquals("Statement.executeQuery() cannot issue statements that do not produce result sets.", exception.getMessage());
}
3. Resolve the Issue
The solution to this exception is straightforward: we must use the correct method for the type of SQL statement we intend to execute.
To illustrate, let’s revisit the example we discussed earlier, in which we attempted to execute an INSERT statement using the executeQuery() method. This time, we’ll correct our approach by using the executeUpdate() method. Afterward, we’ll query the database to confirm that the data has been persisted correctly.
Let’s now inspect the test with a corrected version of the code:
@Test
void givenInsertSql_whenExecuteUpdate_thenAssertUserSaved() throws SQLException {
String insertSql = "INSERT INTO users (username, email) VALUES (?, ?)";
PreparedStatement insertStatement = connection.prepareStatement(insertSql);
insertStatement.setString(1, USERNAME);
insertStatement.setString(2, EMAIl);
insertStatement.executeUpdate();
String selectSql = "SELECT * FROM users WHERE username = ?";
PreparedStatement selectStatement = connection.prepareStatement(selectSql);
selectStatement.setString(1, USERNAME);
ResultSet resultSet = selectStatement.executeQuery();
resultSet.next();
assertEquals(USERNAME, resultSet.getString("username"));
assertEquals(EMAIl, resultSet.getString("email"));
}
Here is a quick overview of the available methods and their purpose:
Method | Purpose |
---|---|
executeQuery() | It’s used to execute SELECT statements that retrieve data from the database. |
executeUpdate() | It’s used to execute DML statements such as INSERT, UPDATE, DELETE, and DDL statements such as CREATE and ALTER. |
execute() | It’s used to execute any SQL statements, usually when the type isn’t determined in advance. |
4. Conclusion
In this article, we’ve explored the uncommon error Cannot issue data manipulation statements with executeQuery() and understood its cause. We also learned the importance of using the correct JDBC methods for specific SQL statements, as each method serves a unique purpose.
As always, full code examples are available over on GitHub.
The post Fix the Exception “Cannot issue data manipulation statements with executeQuery()” first appeared on Baeldung.