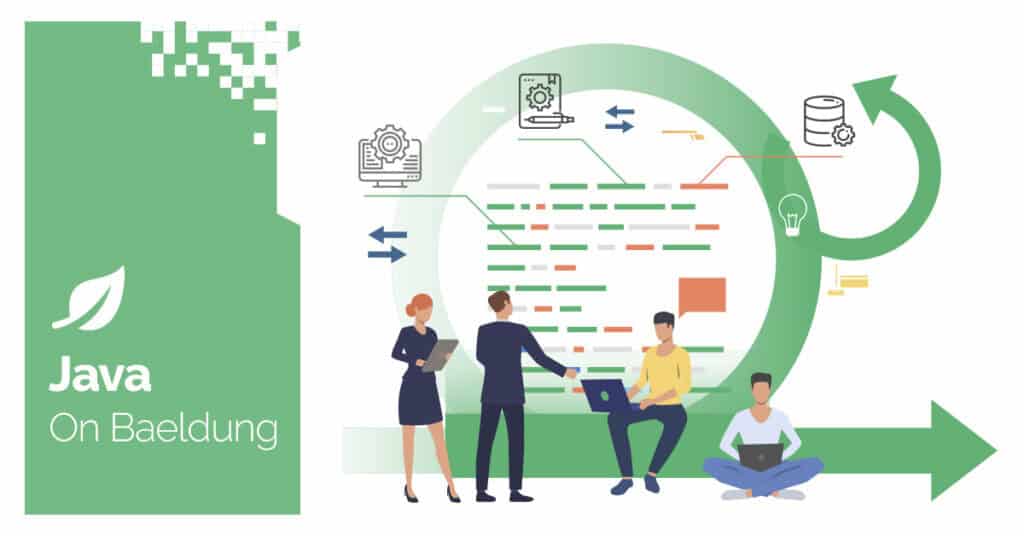
1. Introduction
In this tutorial, we’ll examine predefined properties within Maven.
Properties make build configurations more dynamic, flexible, and reusable. It lets us set up different levels of properties to have default values and then easily override them whenever needed.
Let’s explore these properties in more detail.
2. Maven Predefined Properties
Maven has some handy built-in properties that we can use to streamline our configurations. We can even customize them right in our pom.xml file if needed.
They can also be used in any resource file being processed by the Maven resource plugin’s filtering features, for example, the application.properties file. We just need to wrap them in ${} whenever we use them.
2.1. Usage Example
Let’s see the usage of a property that gives the location for the output directory:
<?xml version="1.0" encoding="UTF-8"?>
<project>
<modelVersion>4.0.0</modelVersion>
<groupId>example.override.properties</groupId>
<artifactId>parameter-maven-plugin</artifactId>
<version>1.0-SNAPSHOT</version>
<packaging>maven-plugin</packaging>
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-plugin-plugin</artifactId>
<version>3.2</version>
<configuration>
<outputDirectory>${project.build.directory}</outputDirectory>
</configuration>
</plugin>
</plugins>
</build>
</project>
The above example shows how we can fetch the default value of the ${project.build.directory}, i.e., src/main/resources.
2.2. Overriding Property Example
Let’s see a property to give a custom build path:
<?xml version="1.0" encoding="UTF-8"?>
<project>
<modelVersion>4.0.0</modelVersion>
<groupId>example.override.properties</groupId>
<artifactId>parameter-maven-plugin</artifactId>
<version>1.0-SNAPSHOT</version>
<packaging>maven-plugin</packaging>
<build>
<directory>src/main/resources/custom</directory>
</build>
</project>
In the above example, we’ve overridden the default value of the property ${project.build.directory} to src/main/resources/custom by specifying the custom value in the hierarchy <project> -> <build> -> <directory>.
3. Project Information Properties
Let’s see some properties used to reference information about the project, such as its configuration, metadata, and build information:
Property | Description |
---|---|
${project.packaging} | This property tells Maven what kind of artifact our project produces. By default, it’s set to “jar”. |
${project.basedir} | This points to the root directory of our project – the same folder where our pom.xml file lives. |
Some properties, like ${project.groupId} and ${project.version}, can inherit default values from the parent POM if not explicitly specified.
4. Project Build Information Properties
Now, let’s dive into some specific properties that deal with where things get built and stored during the build process:
Property | Description |
---|---|
${project.build.directory} | This property acts like a central hub for all the files that are generated during the build process. By default, it’s “target/“. |
${project.build.outputDirectory} | It tells us where Maven puts all the compiled classes for our main application code. The default location is “target/classes“. |
${project.build.testOutputDirectory} | Compiled test classes are placed in “target/test-classes” by default. |
${project.build.sourceDirectory} | This one points to the directory where our main Java source code resides. The default location is “src/main/java“. |
${project.build.testSourceDirectory} | This property tells us where our unit test source code is stored. By default, it’s in “src/test/java“. |
${project.build.resources} | This one specifies the directory containing all our project’s resource files, like configuration files or images. The default location is “src/main/resources“. |
${project.build.testResources} | Similar to the previous one, but specifically for resources used during testing. These are typically located in “src/test/resources” by default. |
${project.build.finalName} | This property determines the name of the final build artifact, like a JAR or WAR file. By default, it combines our project’s artifact ID with the version number, like “${{project.version}“. |
5. System Properties
On top of its own built-in properties, Maven can also tap into the system properties provided by our computer’s Java Virtual Machine (JVM) or those defined when we run a program. These properties are like handy shortcuts to environment-specific details, such as file paths or our operating system:
Property | Description |
---|---|
${java.home} | This one tells us where the JDK or JRE is installed on our system.
It’s generally best to avoid using this property directly. The reason is that different build environments (like CI/CD) might have different JDK locations, which could lead to unexpected issues. A better approach is to use ${env.JAVA_HOME} or create a custom property that explicitly points to our JDK location because it specifically refers to the JAVA_HOME environment variable, which usually points to the root directory of our JDK installation. |
$(java.version} | This property simply tells us the version of Java we’re using. |
${os.name} and ${os.version} | These two properties work together to tell us what operating system we’re on (like Windows or macOS) and its version number. |
${user.dir} | This property tells us the current working directory where we execute Maven. |
6. Maven-Specific Properties
Let’s see the properties that are specific to Maven’s runtime and configuration:
Property | Description |
---|---|
${maven.version} | This property tells us exactly which version of Maven is currently running. |
${settings.localRepository} | This property points to the location of our local Maven repository. By default, it’s stored in ${user.home}/.m2/repository. This is where Maven downloads and stores all our project dependencies. |
${settings.interactiveMode} | This property determines whether Maven can ask us for input during the build process. By default, it’s set to true. |
${settings.offline} | This property controls whether Maven should operate in offline mode. When set to true (which isn’t the default), Maven only uses dependencies that are already available in our local repository. This is useful when we don’t have an active internet connection. |
7. Conclusion
In this article, we explored several built-in properties that Maven provides. Essentially, they allow us to easily tweak and change settings directly within our pom.xml file.
Furthermore, it’s always a good idea to use these properties instead of hardcoding things like paths, values, or any other configurations. This makes our project much more flexible and easier to maintain.
The post Maven Predefined Properties first appeared on Baeldung.