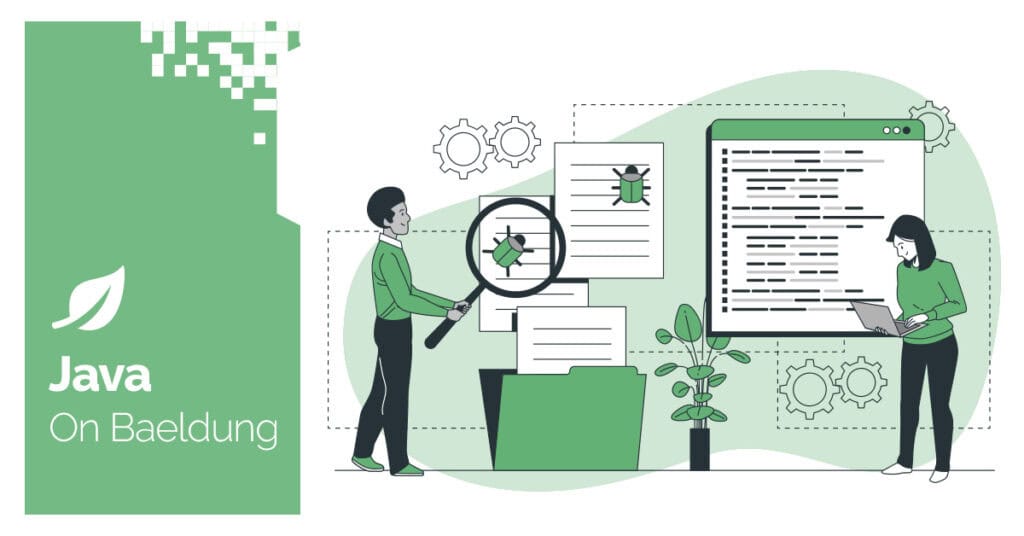
1. Overview
RESTful services are a core component of backend development. Yet, handling HTTP responses can sometimes feel complex. Many developers face challenges when working with response bodies from POST requests in JAX-RS.
In this tutorial, we’ll explore the JAX-RS Client API, focusing on demystifying response handling while providing practical, actionable solutions. Whether a seasoned developer or just starting, it will also equip us with the tools to manage response bodies confidently.
2. JAX-RS Client API & Common Challenges
JAX-RS is a Java API for sending and receiving data in and out of RESTful web services. The client-side API simplifies interactions with REST services, enabling developers to:
- Construct HTTP requests
- Send them to servers
- Process responses with precision and efficiency
Among the key classes in JAX-RS, the Response class is essential. It represents the HTTP response received from a server and provides methods to handle various aspects of the response effectively.
Before going deep into solutions, it’s helpful to identify the common challenges developers face:
- Unexpected response formats: Sometimes, the response format may not match what the client expects, making it difficult to parse or process.
- Empty responses: Servers can occasionally return blank responses, causing issues in processing logic.
- Error handling: Managing HTTP errors is crucial to prevent application crashes or inconsistent data.
3. Working With JAX-RS Client
To effectively work with response bodies in JAX-RS, we need to set up a JAX-RS client. For this example, we’ll use Jersey as the implementation and Jackson for JSON processing.
3.1. Maven Dependencies
First, let’s ensure we have the required Jersey dependencies in our pom.xml file:
<dependency>
<groupId>org.glassfish.jersey.core</groupId>
<artifactId>jersey-client</artifactId>
<version>3.0.4</version>
</dependency>
<dependency>
<groupId>org.glassfish.jersey.media</groupId>
<artifactId>jersey-media-json-jackson</artifactId>
<version>3.0.4</version>
</dependency>
3.2. Creating a Basic JAX-RS Client
Let’s see an example of sending a POST request and reading the response:
public class GenericRestResponse {
private final Logger logger;
private final Client client;
public GenericRestResponse(Client client, Logger logger) {
this.client = client;
this.logger = logger;
}
public GenericRestResponse() {
this(ClientBuilder.newClient(), LoggerFactory.getLogger(GenericRestResponse.class));
}
public void sendRequest(String url, String jsonPayload) {
WebTarget target = client.target(url);
Response response = target.request(MediaType.APPLICATION_JSON)
.post(Entity.entity(jsonPayload, MediaType.APPLICATION_JSON));
try {
if (response.getStatus() == Response.Status.OK.getStatusCode()) {
String responseBody = response.readEntity(String.class);
logger.info("Response Body: " + responseBody);
} else {
logger.error("Failed to get a successful response");
}
} catch (RuntimeException e) {
logger.error("Error processing response", e);
} finally {
response.close();
client.close();
}
}
}
This example demonstrates how to create a complete API interaction workflow. We begin by setting up the REST client, where the Client and WebTarget objects are used to define the API endpoint.
Next, we prepare the request payload by creating a JSON structure that will be sent in the POST request.
The process continues with making the actual POST request, which involves sending the prepared payload to the server and capturing the response. Once we receive the response, we process it by checking if the status is HTTP 200 OK, after which we extract and print the response body.
Finally, we implement proper resource management by closing both the Response and Client objects to ensure efficient resource utilization and prevent memory leaks.
4. Handling Different Content Types
In most web applications, we primarily handle JSON and XML responses. Let’s look at how to work with these different content types.
4.1. JSON Responses
For JSON responses, let’s see how to parse them using Jackson, a library that makes parsing easy:
public class JsonResponse {
private final Logger logger;
private final Client client;
private final String baseUrl;
public JsonResponse(Client client, Logger logger, String baseUrl) {
this.client = client;
this.logger = logger;
this.baseUrl = baseUrl;
}
public User fetchUserData(int userId) {
WebTarget target = client.target(baseUrl);
String jsonPayload = String.format("{\"id\":%d}", userId);
try (Response response = target.request(MediaType.APPLICATION_JSON)
.post(Entity.entity(jsonPayload, MediaType.APPLICATION_JSON))) {
if (response.getStatus() == Response.Status.OK.getStatusCode()) {
return response.readEntity(User.class);
} else {
logger.error("Failed to get user data. Status: {}", response.getStatus());
return null;
}
} catch (Exception e) {
logger.error("Error processing user data", e);
return null;
}
}
}
Here a POST request is sent with a JSON payload. The response body is parsed into a User object using the readEntity() method. This method reads and deserializes the response content directly into the specified class (User) for easy processing. We must ensure the User class is properly annotated so that the JSON mapping works seamlessly with Jackson.
4.2. XML Responses
For XML responses, JAXB is a reliable option:
public class XMLResponse {
private final Logger logger;
private final Client client;
private final String baseUrl;
public XMLResponse(Client client, Logger logger, String baseUrl) {
this.client = client;
this.logger = logger;
this.baseUrl = baseUrl;
}
public Product fetchProductData(int productId) {
WebTarget target = client.target(baseUrl);
String xmlPayload = String.format("%d", productId);
try (Response response = target.request(MediaType.APPLICATION_XML)
.post(Entity.entity(xmlPayload, MediaType.APPLICATION_XML))) {
if (response.getStatus() == Response.Status.OK.getStatusCode()) {
JAXBContext jaxbContext = JAXBContext.newInstance(Product.class);
Unmarshaller unmarshaller = jaxbContext.createUnmarshaller();
return (Product) unmarshaller.unmarshal(response.readEntity(InputStream.class));
} else {
logger.error("Failed to get product data. Status: {}", response.getStatus());
return null;
}
} catch (JAXBException e) {
logger.error("Error unmarshalling product data", e);
return null;
} catch (Exception e) {
logger.error("Error processing product data", e);
return null;
}
}
}
In this example, we send the XML payload as a POST request. The readEntity() method extracts the response as an InputStream, and JAXB’s Unmarshaller class deserializes it into a Product object. To ensure proper XML mapping, we must annotate the Product class with JAXB annotations (@XmlRootElement, @XmlElement, etc.).
5. Error Handling and Response Status
Effective error handling is crucial when managing HTTP responses. We should always verify the response status before reading the body:
if (response.getStatus() != 200) {
logger.error("Error: " + response.getStatus());
}
Implementing proper logging practices is essential to ensuring effective debugging and monitoring of API interactions. This helps us to track request-response flows, identify errors, and improve system observability.
6. Best Practices & Advanced Techniques
Let’s see some best practices when using the JAX-RS API to send or receive requests:
- Optimize performance: We use connection pooling to reduce latency and manage resources effectively.
- Ensure security: We secure API interactions with authentication, encryption, and data validation mechanisms.
- Plan for scalability: We should design clients to handle increased traffic gracefully.
For complex scenarios, we should consider implementing custom message body readers. These are useful for handling custom response types or processing large datasets. Moreover, asynchronous processing enhances responsiveness, especially for long-running requests or streaming data.
- Custom message body readers: Custom message body readers allow us to define how specific/custom data types are deserialized from HTTP responses, providing granular control over the parsing process.
- Asynchronous processing: Asynchronous processing is a feature of JAX-RS that can improve performance, particularly when it’s done for long-running requests or streaming data.
7. Conclusion
In this article, we saw how we can handle HTTP responses effectively and build RESTful applications with the JAX-RS Client API and the best practices. Later, we explored some advanced topics like custom message body readers or asynchronous processing to improve performance.
As always, the full source code for this article is over on GitHub.
The post Read Response Body in JAX-RS Client first appeared on Baeldung.