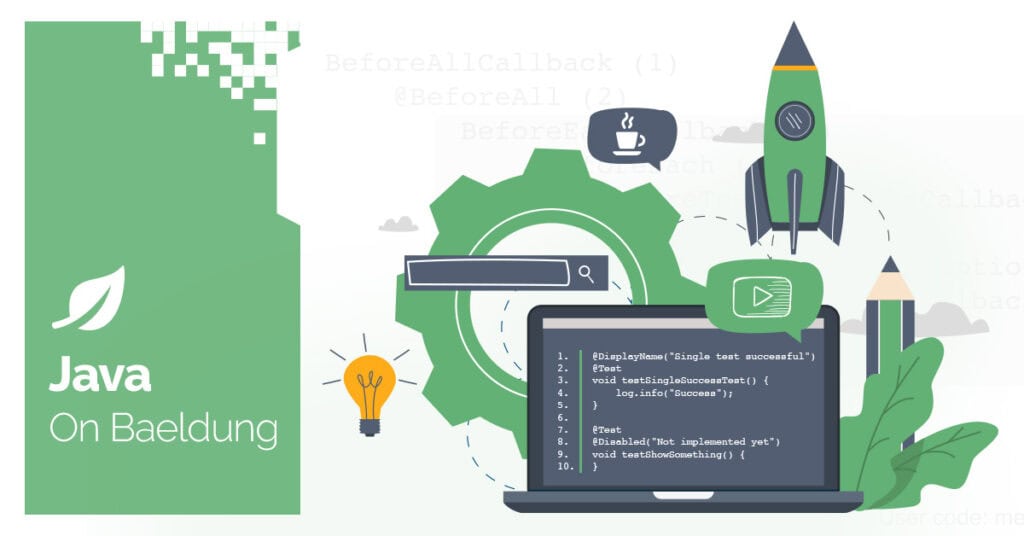
1. Overview
In this tutorial, we’ll learn how to avoid inserting a null value into a map. We’ll focus on the put() method of the Map interface to add a new key-value pair.
2. Using the if Statement
For our example, we’ll have a map and null String variable:
private final Map<Integer, String> map = new HashMap<>();
private final String value = null;
We can avoid inserting null values into map by invoking the put() method only when our variable isn’t null. This can be done through the use of an if statement:
@Test
void givenNullValue_whenUsingIfStatementWithPutMethod_thenObtainMapWithNonNullValues() {
if (value != null) {
map.put(1, value);
}
assertThat(map).isEmpty();
}
As we can see, we avoided inserting null values into our map by wrapping the invocation of the put() method in conditional logic.
3. Using the Optional.ifPresent() Method
Alternatively, we can use the Optional class to conditionally invoke the put() method:
@Test
void givenNullValue_whenUsingOptionalIfPresentWithPutMethod_thenObtainMapWithNonNullValues() {
Optional.ofNullable(value).ifPresent(nonNullValue-> map.put(1, nonNullValue));
assertThat(map).isEmpty();
}
First, we obtain an Optional of our variable using the ofNullable() method. This method returns an Optional with a value if it’s present or an empty Optional if the value is null.
Next, we leverage the ifPresent() method to conditionally invoke the put() method if the Optional has a value. In our case, we have an empty Optional. Thus, the put() method is never invoked protecting our map from the insertion of null values.
4. Using the Stream.filter() Method
If we have a collection as the source of our potentially nullable values, we can use the filter() method to avoid inserting null values into our map. One possible scenario is that we want to create a new map from an existing map but without any null values.
For an existing map that already has a null value in a key-value pair, we can use the filter() method:
@Test
void givenMapWithNullValue_whenUsingStreamFilter_thenObtainMapWithNonNullValues() {
final Map<Integer, String> nullValueMap = new HashMap<>();
nullValueMap.put(1, value);
final Map<Integer, String> mapWithoutNullValues = nullValueMap
.entrySet()
.stream()
.filter(entry -> Objects.nonNull(entry.getValue()))
.collect(Collectors.toMap(Map.Entry::getKey, Map.Entry::getValue));
assertThat(mapWithoutNullValues).isEmpty();
}
First, we obtain a stream of map entries by invoking the stream() method on the Set<Map.Entry> returned by entrySet(). Next, we use the filter() method to remove elements from our stream based on the supplied predicate. Our predicate returns true if the value of the map entry is null. We do this using a method reference for the Objects.nonNull() method.
However, we could have used alternative predicates to achieve the same effect. For example, we could use an if statement as part of a lambda expression:
.filter(entry -> {
if(entry.getValue() != null)
return true;
else
return false;
})
Or, we could use the isPresent() method of the Optional class instead:
.filter(entry -> Optional.ofNullable(entry.getValue()).isPresent())
In any case, we collect the filtered elements into a new map ensuring no null values.
5. Conclusion
In this article, we explored different ways to avoid inserting null values into a map using the put() method. It should be noted that in order to ensure a map can never have null values, other ways to insert key-values into maps must be considered. For example, using constructors for concrete Map classes and methods such as putAll().
As always, the code samples used in this article are available over on GitHub.
The post Putting Value Into Map if Not Null in Java first appeared on Baeldung.