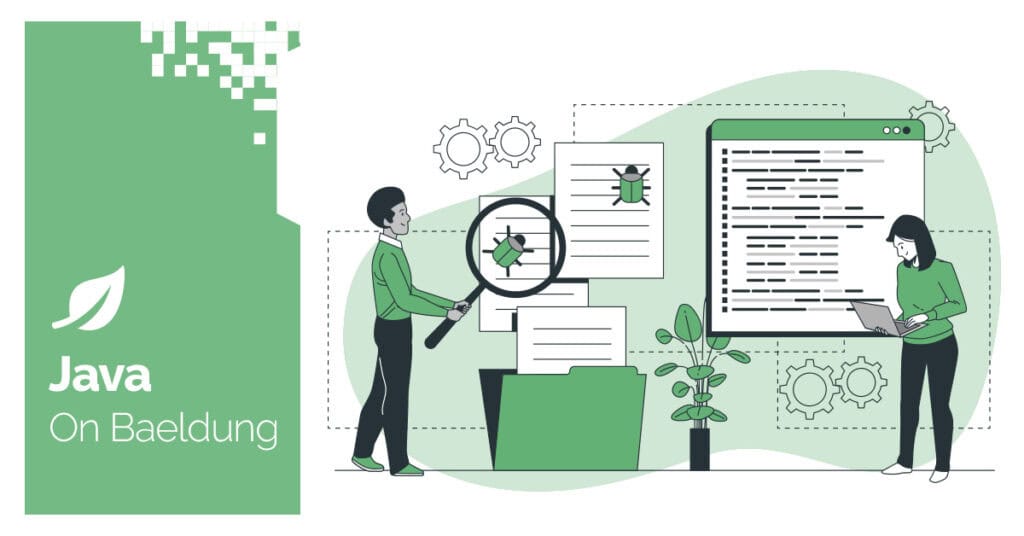
1. Introduction
When writing unit tests with EasyMock, we often need to verify that the methods are called with specific types of arguments. EasyMock provides two main matching methods for this purpose: isA() and anyObject().
While they might seem similar at first glance, they have distinct behaviors and use cases. In this tutorial, we’ll explore the differences between them and learn when to use each.
2. Understanding EasyMock Matchers
Before we start working on the testing examples, let’s reference the EasyMock library. Here’s the dependency we’re going to need in our Maven project:
<dependency>
<groupId>org.easymock</groupId>
<artifactId>easymock</artifactId>
<version>5.5.0</version>
<scope>test</scope>
</dependency>
Now, matchers in EasyMock allow us to define expectations about method parameters without specifying exact values. They’re particularly useful when we care about the type of argument rather than its specific value.
For our examples, let’s create a simple Service interface:
interface Service {
void process(String input);
void handleRequest(Request request);
}
Next, let’s create the two classes we’ll need in our tests:
class Request {
private String type;
Request(String type) {
this.type = type;
}
}
class SpecialRequest extends Request {
SpecialRequest() {
super("special");
}
}
2.1. The isA() Matcher
The isA() matcher verifies that an argument is an instance of a specific class. Furthermore, it also accepts subclasses of the aforementioned class. Its strictness is about the type of argument and null values.
Let’s take a look at this example:
@Test
void whenUsingIsA_thenMatchesTypeAndRejectsNull() {
Service mock = mock(Service.class);
mock.process(isA(String.class));
expectLastCall().times(1);
replay(mock);
mock.process("test");
verify(mock);
}
Initially, we created the mock. Then, we register the expectations (process() and expectLastCall().times()). Next, we activate the mock, replay(mock). Furthermore, we call the desired method, mock.process(“test”). Finally, we perform the check using verify(mock).
In addition, when using isA(), we can also verify behavior with inheritance:
@Test
void whenUsingIsAWithInheritance_thenMatchesSubclass() {
Service mock = mock(Service.class);
mock.handleRequest(isA(Request.class));
expectLastCall().times(2);
replay(mock);
mock.handleRequest(new Request("normal"));
mock.handleRequest(new SpecialRequest()); // SpecialRequest extends Request
verify(mock);
}
Now, let’s go over some key characteristics of isA():
- Performs strict type-checking
- Never matches null values
- Matches subclasses of the specified type
- More suitable for enforcing type safety
If we try to pass null when using isA(), the test fails:
@Test
void whenUsingIsAWithNull_thenFails() {
Service mock = mock(Service.class);
mock.process(isA(String.class));
expectLastCall().times(1);
replay(mock);
assertThrows(AssertionError.class, () -> {
mock.process(null);
verify(mock);
});
}
2.2. The anyObject() Matcher
The anyObject() matcher is more permissive than isA(). Thus, it provides a flexible way to match any object, including null values. This matcher is particularly useful when we don’t care about the specific type or value of an argument.
Now, let’s look at how we can use anyObject():
@Test
void whenUsingAnyObject_thenMatchesNullAndAnyType() {
Service mock = mock(Service.class);
mock.process(anyObject());
expectLastCall().times(2);
replay(mock);
mock.process("test");
mock.process(null);
verify(mock);
}
We can also use anyObject() with a type parameter for better readability:
mock.process(anyObject(String.class));
However, it’s important to note that the type parameter in anyObject(String.class) is mainly for code readability and type inference. Unlike isA(), it doesn’t enforce strict type-checking.
Now, let’s look at some key characteristics of anyObject():
- Accepts any object type
- Matches null values
- Less strict type-checking
- More flexible for general matching
- Can include an optional type parameter for better readability
3. Key Differences
Let’s examine the main differences between these matchers:
isA() | anyObject() | |
---|---|---|
null handling | Never matches null values. Fails test if null is passed | Accepts null values as valid parameters |
Type safety | Enforces strict type checking at runtime | Is type-agnostic and more permissive |
Inheritance | Explicitly verifies the type hierarchy | Accepts any type regardless of inheritance |
When working with EasyMock,we’ll have to choose our matcher based on the testing requirements.
We use isA() when:
- We need to ensure type safety
- null values should be rejected
- We want to explicitly verify type hierarchies
- We test code that should never receive null values
We use anyObject() when:
- We need to accept null values
- Type-checking isn’t critical
- We want more flexible argument-matching
- Testing code that can handle various types of input
4. Conclusion
In this tutorial, we explored the differences between isA() and anyObject() in EasyMock.
First, we looked at how we can use the matchers. Then, we looked at their distinct characteristics. We learned that isA() provides strict type checking and null rejection, while anyObject() offers more flexibility.
Next, we explored their behavior with inheritance and null values through practical examples. Here, the key takeaway is that both matchers serve important, but different purposes: isA() is better when type safety and null prevention are crucial; anyObject() is more suitable when flexibility and null acceptance are needed.
By understanding these differences, we can write more effective and maintainable unit tests that properly verify our code’s behavior.
As always, the code is available over on GitHub.
The post Difference Between isA() and anyObject() in EasyMock first appeared on Baeldung.