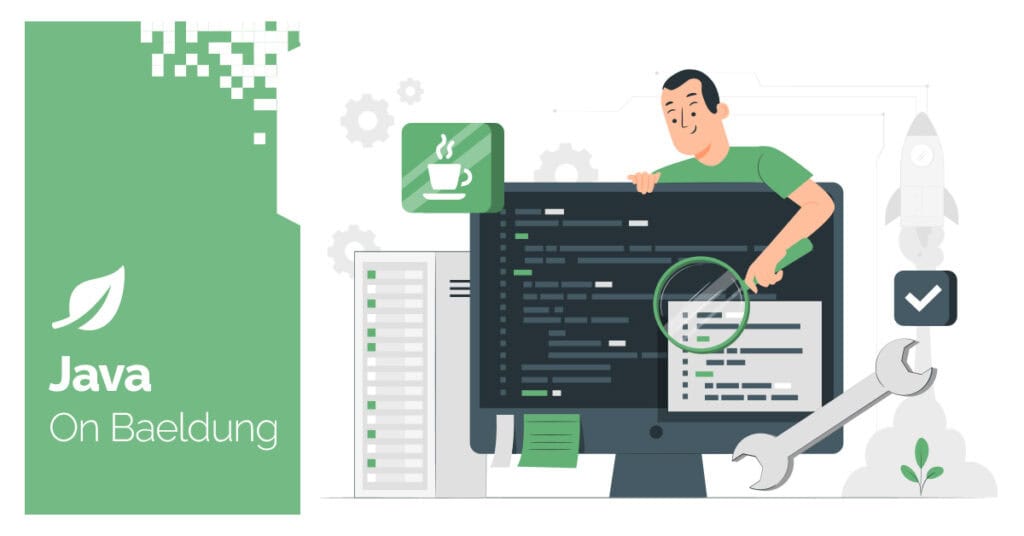
1. Overview
switch statements are commonly used to simplify complex if-else chains. They provide a way to perform different actions based on a single variable’s value.
However, a switch statement in Java traditionally works with specific values such as integers, characters, or enumerations. In recent versions of Java (Java 12 and beyond), the switch statement has evolved to allow for more flexible case matching, but greater-than-or-equal-to conditions are still not directly supported in a traditional switch case structure. This means we cannot directly use >= in a switch statement’s case labels.
In this tutorial, we’ll explore how to work with such conditions, look at workarounds, and learn when and why we might need them.
2. The Challenge With >= In switch Statements
Java’s switch statement cannot handle relational operators such as >=, <=, or !=. It only matches specific, exact values in case labels. Because of this, writing a switch statement with conditions such as “greater than or equal to” isn’t directly possible. For example, the following code is invalid in Java:
int score = 85;
switch (score) {
case >= 90: // Compilation error
System.out.println("Grade: A");
break;
case >= 80: // Compilation error
System.out.println("Grade: B");
break;
default:
System.out.println("Grade: F");
break;
}
This code results in a compilation error because the switch statement expects exact values in each case, not relational conditions. This limitation necessitates the use of alternative approaches to achieve the desired functionality.
3. Alternative Approaches
Since Java doesn’t support relational operators in switch statements, we can use alternative methods to implement conditions like >=. Let’s look at three common approaches.
3.1. Using if-else Statements
Using if-else statements is a straightforward and effective way to handle conditions involving >=. This approach allows for direct comparisons, making the logic easy to follow. Since if-else supports relational operators, it provides flexibility that switch statements lack, especially when working with range-based conditions.
In the example below, we evaluate a student’s score and assign a grade based on predefined thresholds:
String assignGradeUsingIfElse(int score) {
if (score >= 90) {
return "Grade: A";
} else if (score >= 80) {
return "Grade: B";
} else if (score >= 70) {
return "Grade: C";
} else if (score >= 60) {
return "Grade: D";
}
return "Grade: F";
}
This method ensures clarity by processing conditions sequentially and is particularly useful when dealing with a manageable number of cases. Unlike switch statements requiring exact values, the if-else structures allow for greater flexibility and readability.
3.2. Using Ranges With Integer Division
If we want to use a switch statement while handling range-based conditions, we can achieve this by dividing the value into discrete ranges. This method is particularly useful when the ranges are evenly distributed and easy to group.
By dividing the score by 10 for example, we convert it into a more manageable form that allows us to use a switch statement effectively. Here’s an example:
String assignGradeUsingRangesWithIntegerDivision(int score) {
int range = score / 10;
switch (range) {
case 10:
case 9:
return "Grade: A";
case 8:
return "Grade: B";
case 7:
return "Grade: C";
case 6:
return "Grade: D";
default:
return "Grade: F";
}
}
In this approach, the score is divided by 10, effectively grouping scores into categories:
- Scores between 90-100 result in 9 or 10, which maps to “Grade: A“
- Scores between 80-89 result in 8, which maps to “Grade: B“
- Scores between 70-79 result in 7, which maps to “Grade: C“
- Scores between 60-69 result in 6, which maps to “Grade: D“
- Any score below 60 falls into the default case, mapping to “Grade: F“
This method works best when dealing with evenly distributed numeric ranges, making it a viable alternative to if-else statements in cases where a switch statement is preferred.
4. Conclusion
Java’s switch statement doesn’t support relational operators like >=, but there are a few ways to work around this limitation. if-else statements are a straightforward choice for handling such conditions. switch with integer division groups values into ranges, making the switch more flexible.
These approaches help handle range-based conditions effectively while maintaining code clarity within Java’s constraints.
As always, the code in the article is available over on GitHub.
The post Using Greater-Than-Or-Equal-to in a Switch Statement in Java first appeared on Baeldung.