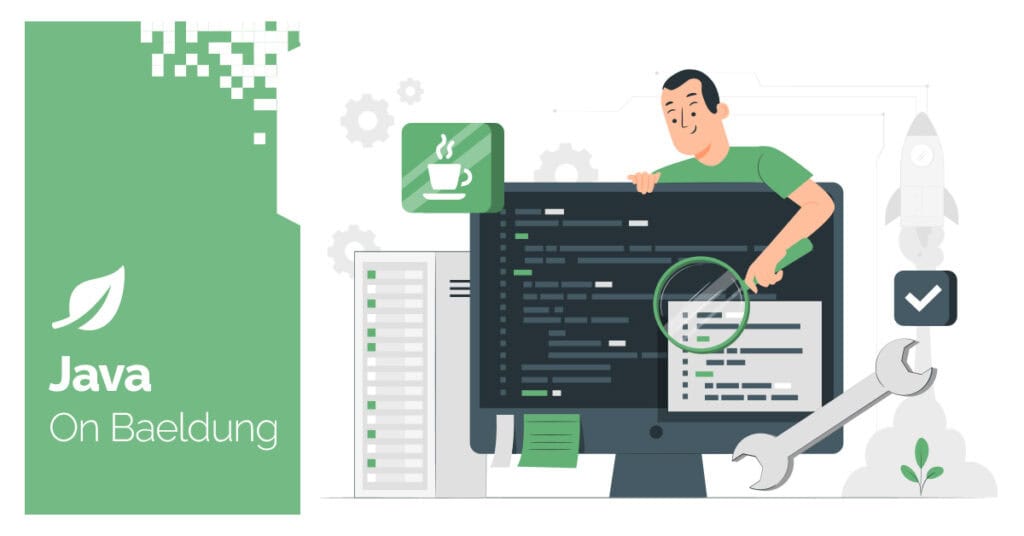
1. Overview
When working with arrays in Java, there are situations where we need to identify whether the current element in an iteration is the last one.
In this tutorial, let’s explore a few common ways to achieve this, each with its advantages depending on the context of our application.
2. Introduction to the Problem
As usual, let’s understand the problem through examples. For example, let’s say we have an array:
String[] simpleArray = { "aa", "bb", "cc" };
Some might think the last element determination while iteration is a simple problem since we can always compare the current element to the last element (array[array.length – 1]). Yes, this approach works for arrays like simpleArray.
However, once the array contains duplicate elements, this approach won’t work anymore, for instance:
static final String[] MY_ARRAY = { "aa", "bb", "cc", "aa", "bb", "cc"};
Now, the last element is “cc”. But we have two “cc” elements in the array. Therefore, checking if the current element equals the last element can result in the wrong result.
So, we need a stable solution to check whether the current element is the last one while iterating an array.
In this tutorial, we’ll address solutions for different iteration scenarios. Also, to demonstrate each approach easily, let’s take MY_ARRAY as the input and use iteration to build this result String:
static final String EXPECTED_RESULT = "aa->bb->cc->aa->bb->cc[END]";
Of course, various ways exist to join array elements into a String with separators. However, our focus is to showcase how to determine if we’ve reached the last iteration.
Additionally, we shouldn’t forget Java has object arrays and primitive arrays. We’ll also cover primitive array scenarios.
3. Checking the Indexes in Loops
A straightforward method to check if an element is the last in an array is to use a traditional index-based for loop. This approach gives us direct access to each element’s index, which can be compared to the last element’s index in the array to determine if we’re at the last element:
int lastIndex = MY_ARRAY.length - 1;
StringBuilder sb = new StringBuilder();
for (int i = 0; i < MY_ARRAY.length; i++) {
sb.append(MY_ARRAY[i]);
if (i == lastIndex) {
sb.append("[END]");
} else {
sb.append("->");
}
}
assertEquals(EXPECTED_RESULT, sb.toString());
In this example, we first get the last element’s index (lastIndex) in the array. We compare the loop variable i to lastIndex on each iteration to determine if we’ve reached the last element. Then, we can choose the corresponding separator.
Since this approach checks array indexes, it works for object and primitive arrays.
4. Using For-Each Loop with an External Counter
Sometimes, we prefer using a for-each loop, for its simplicity and readability. However, it doesn’t provide direct access to the index. But we can create an external counter to keep track of our position in the array:
int counter = 0;
StringBuilder sb = new StringBuilder();
for (String element : MY_ARRAY) {
sb.append(element);
if (++counter == MY_ARRAY.length) {
sb.append("[END]");
} else {
sb.append("->");
}
}
assertEquals(EXPECTED_RESULT, sb.toString());
In this example, we manually maintain a counter variable that starts at 0 and increments with each iteration. We also check if the counter has reached the given array’s length on each iteration.
The external counter approach works similarly to the index-based for loop. Therefore, it also works for primitive arrays.
5. Converting the Array to an Iterable
Java’s Iterator allows us to iterate data collections conveniently. Furthermore, it provides the hasNext() method, which is ideal to check if the current element is the last one in the collection.
However, arrays don’t implement the Iterable interface in Java. We know Iterator is available in Iterable or Stream instances. Therefore, we can convert the array to an Iterable or Stream to obtain an Iterator.
5.1. Object Arrays
List implements the Iterable interface. So, let’s can convert an object array to a List and get its Iterator:
Iterator<String> it = Arrays.asList(MY_ARRAY).iterator();
StringBuilder sb = new StringBuilder();
while (it.hasNext()) {
sb.append(it.next());
if (it.hasNext()) {
sb.append("[END]");
} else {
sb.append("->");
}
}
assertEquals(EXPECTED_RESULT, sb.toString());
In this example, we use Arrays.asList() to convert our String array to a List<String>.
Alternatively, we can leverage the Stream API to convert an object array to a Stream and obtain an Iterator:
Iterator<String> it = Arrays.stream(MY_ARRAY).iterator();
StringBuilder sb = new StringBuilder();
while (it.hasNext()) {
sb.append(it.next());
if (it.hasNext()) {
sb.append("[END]");
} else {
sb.append("->");
}
}
assertEquals(EXPECTED_RESULT, sb.toString());
As the code above shows, we use Arrays.stream() to get a Stream<String> from the input String array.
5.2. Primitive Arrays
Let’s first create an int[] array as an example and the expected String result:
static final int[] INT_ARRAY = { 1, 2, 3, 1, 2, 3 };
static final String EXPECTED_INT_ARRAY_RESULT = "1->2->3->1->2->3[END]";
Stream API offers three commonly used primitive types of streams: IntStream, LongStream, and DoubleStream. So, if we want to use an Iterator to iterate an int[], long[], or double[], we can easily convert the primitive array to a Stream, for example:
Iterator<Integer> it = IntStream.of(INT_ARRAY).iterator();
StringBuilder sb = new StringBuilder();
while (it.hasNext()) {
sb.append(it.next());
if (it.hasNext()) {
sb.append("[END]");
} else {
sb.append("->");
}
}
assertEquals(EXPECTED_INT_ARRAY_RESULT, sb.toString());
Alternatively, we can still use Arrays.stream() to get a primitive type Stream from an int[], long[], or double[]:
public static IntStream stream(int[] array)
public static LongStream stream(long[] array)
public static DoubleStream stream(double[] array)
If our primitive array isn’t one of int[], long[], or double[], we can convert it to a List of its wrapper type, for example, converting a char[] to a List<Character>. Then, we can use an Iterator to iterate the List.
However, when we perform the primitive array to List conversion, we must walk through the array. Thus, we iterate the array to obtain the List and then iterate the List again for the actual work. Therefore, it’s not an optimal approach to convert a primitive array to a List just for using an Iterator to iterate it.
Next, let’s see how we can use an Iterator to iterate primitive arrays other than int[], long[], or double[].
6. Creating a Custom Iterator
We’ve seen that it’s handy to iterate an array and determine the last iteration using an Iterator. Also, we’ve discussed that obtaining an Iterator of a primitive array by converting the array to List isn’t an optimal approach. Instead, we can implement a custom Iterator for primitive arrays to gain Iterator‘s benefits without creating intermediate List or Stream objects.
First, let’s prepare a char[] array as our input and the expected String result:
static final char[] CHAR_ARRAY = { 'a', 'b', 'c', 'a', 'b', 'c' };
static final String EXPECTED_CHAR_ARRAY_RESULT = "a->b->c->a->b->c[END]";
Next, let’s create a custom Iterator for char[] arrays:
class CharArrayIterator implements Iterator<Character> {
private final char[] theArray;
private int currentIndex = 0;
public static CharArrayIterator of(char[] array) {
return new CharArrayIterator(array);
}
private CharArrayIterator(char[] array) {
theArray = array;
}
@Override
public boolean hasNext() {
return currentIndex < theArray.length;
}
@Override
public Character next() {
return theArray[currentIndex++];
}
}
As the code shows, the CharArrayIterator class implements the Iterator interface. It holds the char[] array as an internal variable. Next to the array variable, we define currentIndex to track the current index position. It’s worth mentioning that autoboxing (char -> Character) happens in the next() method.
Next, let’s see how to use our CharArrayIterator:
Iterator<Character> it = CharArrayIterator.of(CHAR_ARRAY);
StringBuilder sb = new StringBuilder();
while (it.hasNext()) {
sb.append(it.next());
if (it.hasNext()) {
sb.append("->");
} else {
sb.append("[END]");
}
}
assertEquals(EXPECTED_CHAR_ARRAY_RESULT, sb.toString());
If we need custom Iterators for other primitive arrays, we must create similar classes.
7. Conclusion
In this article, we’ve explored how to determine if an element is the last one while iterating over an array in different iteration scenarios. We’ve also discussed the solutions for both object and primitive array cases.
As always, the complete source code for the examples is available over on GitHub.